Generated by Contentify AI
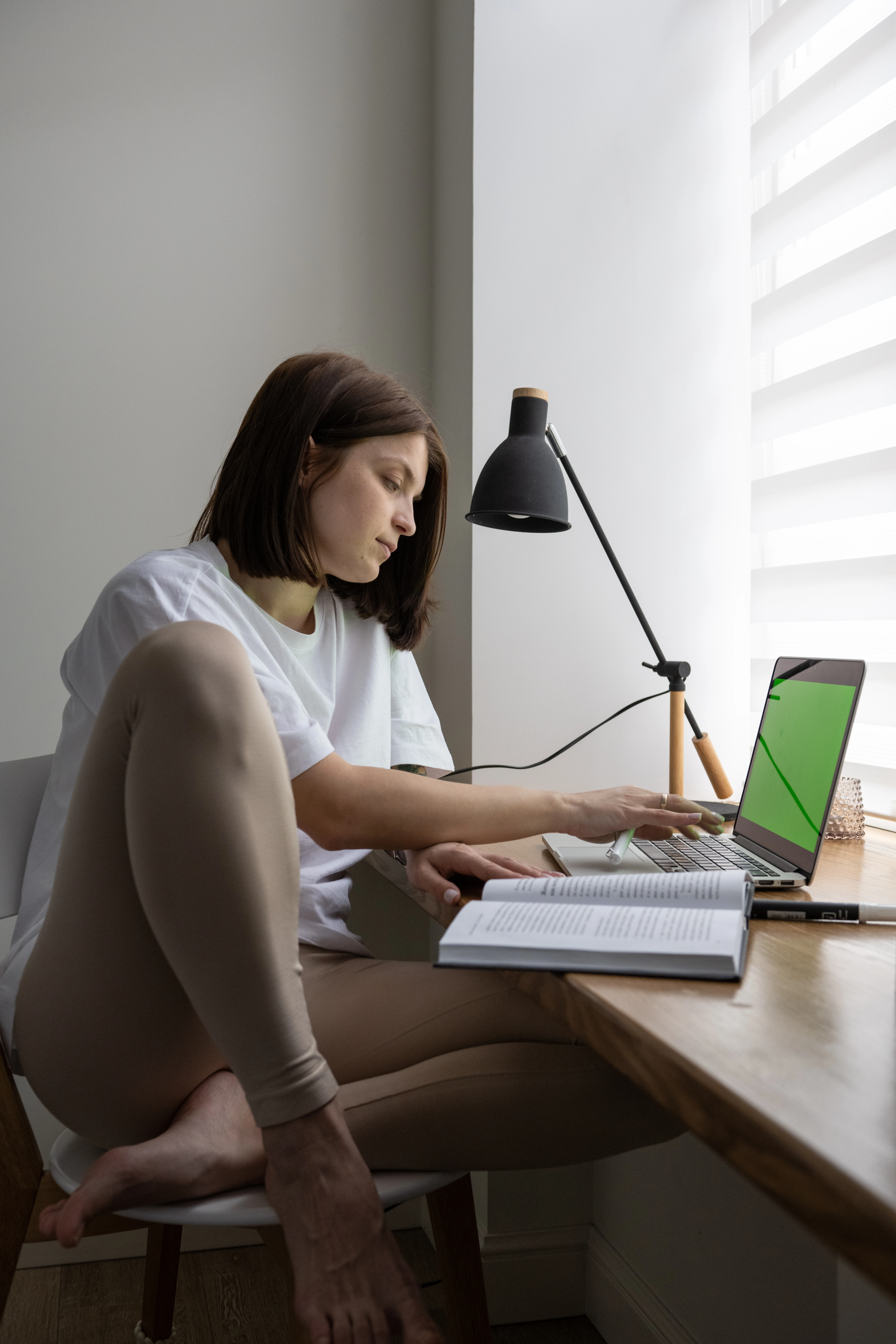
Introduction
jQuery is a widely used JavaScript library that simplifies the process of creating interactive and dynamic web pages. While it offers numerous benefits, developers may encounter issues and errors while working with jQuery. This is where troubleshooting and debugging skills become essential. Troubleshooting and debugging in jQuery involves identifying and resolving issues such as incorrect selectors, syntax errors, and unexpected behavior. By understanding common problems and employing effective debugging techniques, developers can efficiently troubleshoot and debug their jQuery code, ensuring smooth and error-free functionality. In this section, we will explore various strategies and tools that can aid in troubleshooting and debugging in jQuery, helping developers overcome challenges and deliver robust web applications.
Common jQuery Errors
Troubleshooting and debugging are crucial skills for developers working with jQuery. While jQuery simplifies the process of creating interactive web pages, it is not immune to errors. By understanding common jQuery errors and employing effective debugging techniques, developers can efficiently identify and resolve issues.
One common issue is incorrect selectors. Selectors are used to target specific elements on a webpage, but if a selector is written incorrectly, it will fail to find the desired element. Developers can use browser developer tools to inspect the HTML structure and verify that the selector is targeting the correct element.
Syntax errors are another common problem in jQuery. Small mistakes like missing parentheses or semicolons can cause the code to break. It is essential to review the code carefully and check for any syntax errors. Additionally, using a code editor with syntax highlighting can help identify any mistakes.
Unexpected behavior is also a typical challenge when working with jQuery. Sometimes, a function or event handler may not work as expected, resulting in incorrect or non-functional behavior. Developers can use console.log statements to inspect variables and identify where the code is not behaving as intended. By isolating the problem area, they can then debug and troubleshoot the issue effectively.
In conclusion, troubleshooting and debugging in jQuery is essential for maintaining error-free functionality in web applications. By understanding common errors, reviewing syntax, and using debugging tools, developers can quickly identify and resolve issues, ensuring a seamless user experience.
Handling AJAX Errors
Troubleshooting and debugging are crucial skills for developers working with jQuery. While jQuery simplifies the process of creating interactive web pages, it is not immune to errors. Common issues that developers may encounter include incorrect selectors, syntax errors, and unexpected behavior.
One of the most common problems is incorrect selectors. Selectors are used to target specific elements on a webpage, but if a selector is written incorrectly, it will fail to find the desired element. Developers can utilize browser developer tools to inspect the HTML structure and verify that the selector is targeting the correct element.
Syntax errors are another frequent challenge. Small mistakes like missing parentheses or semicolons can cause the code to break. It is crucial to review the code carefully and check for any syntax errors. Additionally, using a code editor with syntax highlighting can help identify any mistakes.
Unexpected behavior is also a typical issue when working with jQuery. Sometimes, a function or event handler may not work as expected, resulting in incorrect or non-functional behavior. Developers can use console.log statements to inspect variables and identify where the code is not behaving as intended. By isolating the problem area, they can then debug and troubleshoot the issue effectively.
In conclusion, troubleshooting and debugging in jQuery are essential for maintaining error-free functionality in web applications. By understanding common errors and employing effective debugging techniques, developers can quickly identify and resolve issues, ensuring a seamless user experience.
Debugging jQuery Code
Debugging jQuery Code
Troubleshooting and debugging in jQuery are essential skills for developers to ensure smooth and error-free functionality in web applications. While working with jQuery, developers may encounter various issues, such as incorrect selectors, syntax errors, and unexpected behavior. These issues can hinder the proper functioning of the code and require careful debugging.
One common challenge is dealing with incorrect selectors. Selectors are used to target specific elements on a webpage, but if a selector is written incorrectly, it will fail to find the desired element. To troubleshoot this issue, developers can use browser developer tools to inspect the HTML structure and validate that the selector is accurately targeting the element.
Syntax errors are another frequent problem when working with jQuery. Small mistakes like missing parentheses or semicolons can cause the code to break. To resolve syntax errors, developers should carefully review their code and use a code editor with syntax highlighting to identify and fix any mistakes.
Another issue that developers may face is unexpected behavior in their jQuery code. Functions or event handlers may not work as intended, resulting in incorrect or non-functional behavior. One effective way to troubleshoot this issue is by using console.log statements to inspect variables and identify where the code is not behaving as expected. By isolating the problem area, developers can then debug and resolve the issue promptly.
In summary, troubleshooting and debugging in jQuery are vital skills for developers. By addressing common issues such as incorrect selectors, syntax errors, and unexpected behavior, developers can ensure that their jQuery code functions correctly and delivers a seamless user experience. With careful debugging and troubleshooting techniques, developers can overcome challenges and deliver robust web applications.
Using Browser Developer Tools
Using Browser Developer Tools
When it comes to troubleshooting and debugging in jQuery, one valuable tool at a developer’s disposal is the browser developer tools. These tools, such as the Chrome DevTools, provide a range of features that can help identify and resolve issues in jQuery code.
One key feature of browser developer tools is the ability to inspect the HTML structure of a webpage. This can be especially useful when dealing with incorrect selectors in jQuery. By inspecting the elements on the page, developers can verify if their selectors are targeting the right elements and make any necessary adjustments.
Another useful feature is the console, which allows developers to log and track messages, variables, and errors directly in the browser. By strategically placing console.log statements in their jQuery code, developers can get a better understanding of how the code is executing and identify any unexpected behavior.
The browser developer tools also provide a JavaScript debugger, which allows developers to set breakpoints and step through their code line by line. This feature is particularly helpful when debugging complex jQuery functions or event handlers. By observing the code execution in real-time, developers can pinpoint and fix any issues effectively.
In conclusion, browser developer tools are a valuable resource for troubleshooting and debugging in jQuery. By utilizing features such as inspecting HTML structure, logging messages in the console, and using the JavaScript debugger, developers can identify and resolve issues efficiently. These tools play a crucial role in ensuring the smooth and error-free functionality of jQuery code, ultimately delivering a seamless user experience.
Using Console.log()
When working with jQuery, developers may encounter various challenges that require troubleshooting and debugging. These issues can range from incorrect selectors and syntax errors to unexpected behavior in the code. Fortunately, there are several effective strategies and tools available to aid in the debugging process. One commonly used technique is the use of console.log(). By strategically placing console.log() statements in the code, developers can output specific values and variables to the browser console. This allows them to inspect and track the execution of the code, helping identify any issues or unexpected behaviors. Additionally, console.log() can be used to verify the values of variables, ensuring they hold the expected data. By utilizing console.log() effectively, developers can gain valuable insights into their jQuery code, making it easier to locate and resolve any problems that may arise.
Debugging with Breakpoints
Troubleshooting and debugging are essential skills for developers working with jQuery. As with any programming language or framework, issues can arise while working with jQuery code. These issues can range from syntax errors and incorrect selectors to unexpected behavior in the code. The key to efficiently resolving these issues lies in the ability to effectively troubleshoot and debug. One useful technique for troubleshooting jQuery code is the strategic use of breakpoints. Breakpoints allow developers to pause the execution of their code at specific points, allowing them to inspect variables, step through the code, and identify any issues or unexpected behaviors. By utilizing breakpoints in conjunction with browser developer tools, developers can gain valuable insights into the flow of their code and pinpoint the exact location where problems occur. This targeted approach to debugging enables developers to isolate and fix issues quickly and efficiently, ensuring the smooth and error-free functionality of their jQuery applications. Troubleshooting and debugging in jQuery is a vital skill set for developers, enabling them to deliver robust and seamless web applications that meet the needs and expectations of users.
Using jQuery Debugging Tools
Using jQuery Debugging Tools
When it comes to troubleshooting and debugging in jQuery, developers have access to a variety of helpful tools. These tools enable developers to identify and fix issues in their code efficiently. By utilizing these debugging tools, developers can streamline the development process and ensure the smooth functionality of their jQuery applications.
One useful debugging tool is the browser developer tools such as Chrome DevTools. These tools provide a range of features that can aid in troubleshooting jQuery code. For example, developers can inspect the HTML structure of a webpage to verify if selectors are correctly targeting the desired elements. Additionally, the browser console allows developers to log messages and track the execution of their code, helping them identify any unexpected behavior or errors.
Another powerful debugging tool is the JavaScript debugger, available in browser developer tools. This tool enables developers to set breakpoints in their code, allowing them to pause the execution and examine variables and the flow of the code. By stepping through the code, developers can pinpoint the exact location of issues and make necessary adjustments.
Additionally, there are various jQuery-specific debugging tools available that offer specific features for troubleshooting jQuery code. These tools provide additional functionality and insights specifically tailored for jQuery development.
In conclusion, troubleshooting and debugging in jQuery are crucial skills for developers. By utilizing debugging tools such as browser developer tools and jQuery-specific tools, developers can efficiently identify and resolve issues in their code. These tools empower developers to deliver robust and error-free jQuery applications, providing a seamless user experience.
Troubleshooting Performance Issues
Troubleshooting and debugging are essential skills for developers working with jQuery. These skills enable developers to identify and resolve issues that may arise during the development process. By effectively troubleshooting and debugging their code, developers can ensure the smooth functionality of their jQuery applications.
One common challenge in troubleshooting jQuery code is dealing with incorrect selectors. Selectors are used to target specific elements on a webpage, and if a selector is written incorrectly, it will fail to find the desired element. To overcome this issue, developers can utilize browser developer tools to inspect the HTML structure and validate that the selector is accurately targeting the element.
Syntax errors are another common issue that developers may encounter. Small mistakes like missing parentheses or semicolons can cause the code to break. Reviewing the code carefully and using a code editor with syntax highlighting can help identify and fix any syntax errors.
Unexpected behavior is also a typical challenge when working with jQuery. Functions or event handlers may not work as expected, resulting in incorrect or non-functional behavior. By strategically incorporating console.log statements in the code, developers can inspect variables and track the execution of the code. This allows them to identify the specific area where the code is not behaving as intended, enabling them to debug and troubleshoot the issue effectively.
By employing effective troubleshooting and debugging techniques, developers can efficiently identify and resolve issues in their jQuery code. This ensures the smooth and error-free functionality of their applications, delivering a seamless user experience. Troubleshooting and debugging in jQuery are essential skills that empower developers to create robust and reliable web applications.
Conclusion
Troubleshooting and debugging are crucial skills for developers working with jQuery. Despite its advantages, developers may encounter issues and errors while working with jQuery code. These challenges can range from incorrect selectors and syntax errors to unexpected behavior. To overcome these obstacles, developers need to employ effective troubleshooting and debugging techniques. By identifying common problems and utilizing debugging tools, developers can efficiently troubleshoot and debug their jQuery code, ensuring smooth and error-free functionality. Common issues include incorrect selectors, which can be resolved by inspecting the HTML structure and validating the selector. Syntax errors can be caught by reviewing the code carefully and using a code editor with syntax highlighting. Unexpected behavior can be addressed by strategically incorporating console.log statements to track the code’s execution and identify the problematic area. By utilizing these techniques, developers can deliver robust web applications that provide a seamless user experience. Troubleshooting and debugging in jQuery are essential skills for developers to overcome challenges and deliver high-quality code.