Generated by Contentify AI
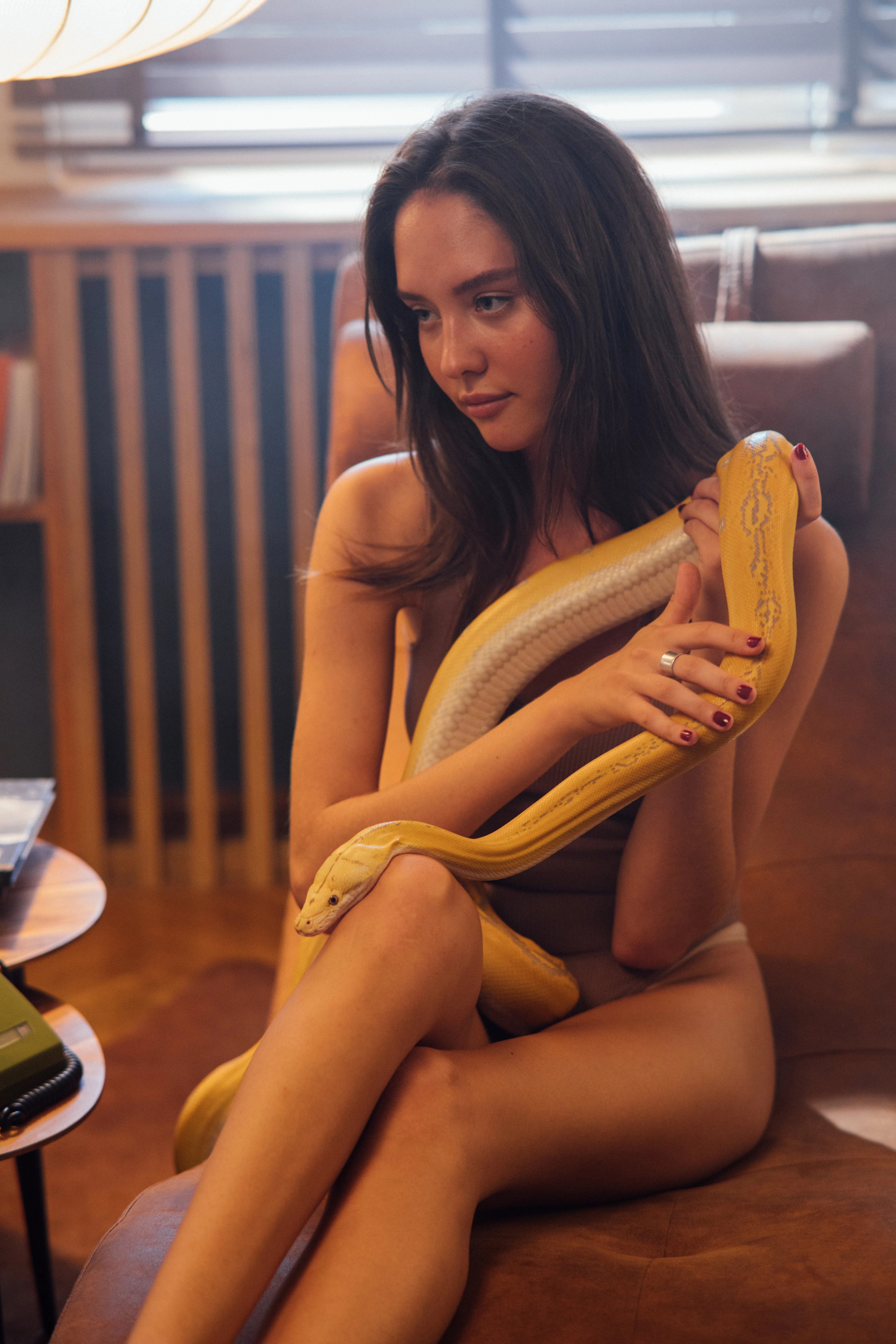
Introduction
Python for loops are a powerful tool for performing repetitive tasks on collections of data, such as lists and tuples. By using a for loop, you can iterate through each item in a list or tuple and perform operations on them. This allows for efficient and streamlined data processing. In this section, we will explore how to use Python for loops to iterate through lists and tuples, and showcase their versatility and usefulness in programming. So, let’s dive in and see how Python for loops can improve your data manipulation skills.
What are loops?
Loops are an essential concept in programming that allow you to repeat a block of code multiple times. In Python, one type of loop is the for loop. The for loop is particularly useful when working with collections of data, such as lists and tuples.
With a for loop, you can iterate through each item in a list or tuple and perform operations on them. This means that you can easily apply the same code to each item, without having to write repetitive code.
For example, let’s say you have a list of numbers and you want to multiply each number by 2. Instead of manually multiplying each number, you can use a for loop to iterate through the list and perform the multiplication operation on each item.
The syntax for a for loop looks like this:
“`
for item in collection:
# code to be executed for each item
“`
In this case, `item` represents the current item being iterated over, and `collection` is the list or tuple being iterated through.
By using the power of for loops, you can easily iterate through lists and tuples, perform operations on each item, and streamline your data manipulation tasks. Python for loops provide a concise and efficient way to process collections of data, making them an essential tool for any programmer.
Introduction to for loops
In Python, for loops are a crucial feature for iterating through collections of data such as lists and tuples. These loops allow you to repeatedly execute a block of code for each item in the collection. By leveraging for loops, you can efficiently process and manipulate data without having to write repetitive code. The syntax for a for loop is simple: for item in collection. With each iteration, you can perform specific operations on the current item. This versatility makes Python for loops an invaluable tool for programmers, enabling them to iterate through lists and tuples effortlessly. By understanding how to utilize for loops effectively, you can enhance your data processing capabilities and streamline your programming tasks.
Iterating through lists
Python for loops provide a powerful mechanism for iterating through collections of data, such as lists and tuples. With a for loop, you can easily traverse each item in the collection and perform operations on them. This makes it incredibly convenient for processing and manipulating data in an efficient manner.
When iterating through a list or tuple using a for loop, you can access each item individually and execute specific code for each iteration. This allows you to perform repetitive tasks on the elements of the collection without writing redundant code. Whether you need to perform calculations, modify the values, or extract specific information, Python for loops simplify the process by automating the iteration.
The syntax for a for loop is straightforward: `for item in collection:`. This means that for each item in the collection, the code inside the loop will be executed. You can access the current item using the variable specified in the loop declaration. This way, you can apply the necessary operations to each item, making it an ideal tool for data manipulation.
Iterating through lists and tuples in Python using for loops can greatly improve your code’s readability and maintainability. It allows you to focus on the logic of the operations you need to perform on each item, rather than worrying about the mechanics of iteration. By leveraging the power of for loops, you can efficiently process and transform your data, making your code more elegant and effective.
Iterating through tuples
Iterating through tuples with Python for loops is a valuable technique for efficiently processing and manipulating data. Tuples are immutable collections that can store multiple elements. With a for loop, you can easily iterate through each item in a tuple and perform operations on them, just like with lists. Whether you need to extract specific information, perform calculations, or modify the values, Python for loops simplify the process by automating the iteration. By accessing each item individually, you can apply the necessary operations and streamline your data processing tasks. The versatile syntax and functionality of Python for loops make them an essential tool for programmers working with both lists and tuples.
Using conditional statements in for loops
Using conditional statements in for loops
Python for loops: Iterating through lists and tuples allows you to not only iterate through each item but also apply conditional statements to perform specific operations based on certain criteria. By combining for loops with conditional statements, you can add logic and control to your data processing tasks.
With conditional statements like if, else, and elif, you can check the values of the items in the collection and execute different code blocks accordingly. This allows you to selectively perform actions based on specific conditions. For example, you can iterate through a list of numbers and only perform an operation on the items that meet a certain criteria, such as being greater than a certain threshold.
The syntax for using conditional statements in for loops is straightforward. After the iteration line, you can add a block of code indented underneath the loop that contains your conditional statements. This code will only execute if the condition is met for the current item.
By combining conditional statements with for loops, you can enhance the flexibility and control of your data processing tasks. This allows you to handle different scenarios and perform specific operations based on the values in the collection. Whether you need to filter, transform, or extract data, Python for loops with conditional statements provide a powerful toolset for efficient and precise data manipulation.
Using range() function with for loops
Using the range() function with for loops in Python allows for precise control over the iterations. This function generates a sequence of numbers that can be used as the basis for iterating through lists and tuples. By specifying the starting point, ending point, and step size, you can customize the range to fit your specific needs. For example, using the range() function with a for loop makes it easy to iterate through every other item in a list or to perform calculations on a specific range of values. This level of control enhances the flexibility and efficiency of your data processing tasks. By leveraging the power of the range() function in combination with Python for loops, you can easily manipulate and analyze data in lists and tuples, making it an essential technique for any programmer.
Nested for loops
Nested for loops provide a powerful technique for performing complex iterations through lists and tuples in Python. By using multiple for loops within each other, you can iterate through nested collections and perform operations on each item. This allows for the traversal of multidimensional data structures, such as matrices or nested lists. With each iteration, you can access and manipulate individual items at each level of nesting. This versatility makes nested for loops an invaluable tool for solving problems that require nested data processing. Whether you need to search for specific values, perform calculations, or generate combinations of items, nested for loops offer a concise and efficient solution. By mastering the art of nested for loops, you can unlock the full potential of Python for loop iteration and tackle even the most complex data manipulation tasks.
Faster alternatives to traditional for loops
Faster alternatives to traditional for loops
While Python for loops are a convenient and effective way to iterate through lists and tuples, there are times when you may need a faster alternative. Traditional for loops can sometimes be slower, especially when dealing with large datasets or complex calculations. Fortunately, Python offers several alternatives that can significantly improve the performance of your code.
One popular alternative is to use list comprehension. List comprehension allows you to create new lists by applying operations to each item in an existing list. It combines the iteration process and operation into a single concise statement. This can save both time and memory by avoiding the need to create intermediate lists and reducing the number of iterations.
Another option is to use the map() function. The map() function applies a given operation to each item in a list or tuple and returns a new iterable object. This can be especially useful when you need to apply a function to every item without explicitly writing a for loop.
Additionally, the numpy library provides a powerful alternative for handling large numerical datasets. The numpy library offers efficient array operations and supports vectorized operations that can be faster than traditional for loops. By leveraging numpy’s capabilities, you can perform calculations on arrays with improved performance and readability.
In summary, while Python for loops are versatile and widely used for iterating through lists and tuples, there are faster alternatives available. List comprehension, the map() function, and numpy library are just a few examples of the options you can explore to optimize your code and improve its performance. By choosing the right alternative based on your specific requirements, you can enhance the efficiency of your data processing tasks.
Conclusion
Conclusion
Python for loops offer a versatile and efficient way to iterate through lists and tuples. By using for loops, you can easily traverse each item in a collection and perform operations on them. Whether you need to manipulate data, extract specific information, or apply conditional statements, for loops provide a streamlined solution. They allow for concise and readable code, enhancing the overall maintainability of your program. Additionally, by utilizing range() functions, conditional statements, and nested for loops, you can further enhance the capabilities of for loops and handle more complex data processing tasks. Furthermore, there are faster alternatives to traditional for loops, such as list comprehension, the map() function, and the numpy library, which can significantly improve performance when dealing with large datasets or complex calculations. Python for loops are an essential tool for any programmer looking to iterate through and manipulate lists and tuples efficiently.