Generated by Contentify AI
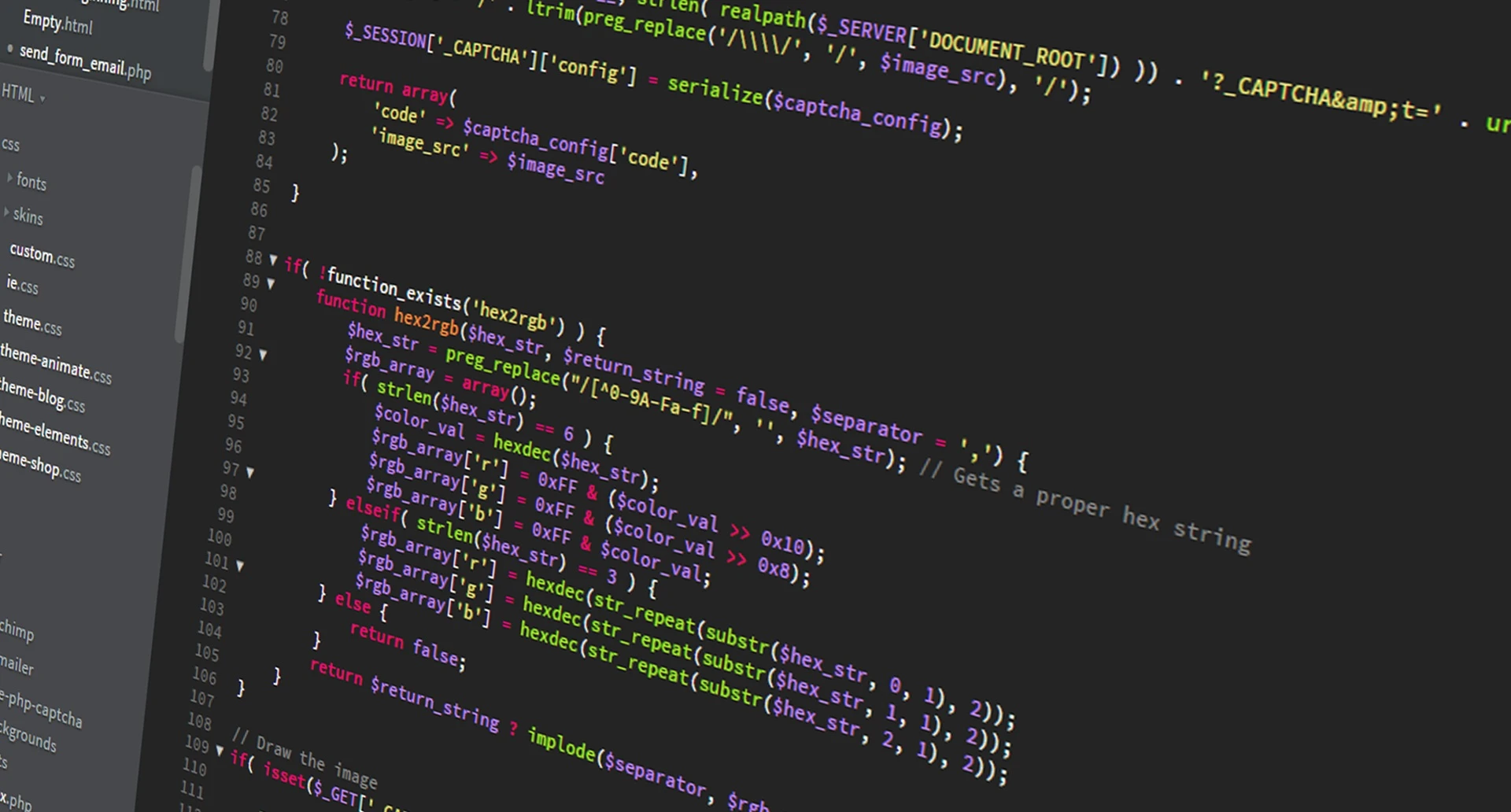
Introduction
PHP is a widely used programming language for developing web applications. However, as your codebase grows, you may encounter performance issues that can impact the user experience. In this section, we will explore various techniques for improving the performance of your PHP code. By implementing these best practices, you can optimize your code, reduce load times, and provide a smoother experience for your users. From optimizing database queries to caching, we will cover everything you need to know to enhance the performance of your PHP code. So let’s dive in and learn how to make your PHP applications faster and more efficient.
Understanding PHP Performance
Understanding PHP Performance is crucial for improving the overall efficiency and responsiveness of your web applications. By familiarizing yourself with the factors that impact PHP performance, you can identify bottlenecks and implement targeted optimizations.
One key aspect of PHP performance is the efficient use of variables and data structures. Avoid using unnecessary variables and arrays, as they consume memory and processing power. Additionally, consider the appropriate data types for your variables, as using the correct ones can have a significant impact on performance.
Another important area to focus on is database optimization. By writing efficient SQL queries and properly indexing your database tables, you can significantly improve the speed of data retrieval. Regularly analyzing and optimizing your database can lead to substantial performance gains.
Caching is another effective technique for enhancing PHP performance. By temporarily storing frequently accessed data in memory or disk-based caches, you can reduce the time spent on expensive computations or database queries. Implementing caching mechanisms such as opcode caching or utilizing tools like Redis or Memcached can greatly enhance the speed of your PHP code.
Furthermore, optimizing code execution by minimizing I/O operations and reducing network latency can greatly improve performance. Consider using asynchronous programming techniques to handle time-consuming tasks without blocking the main execution flow. Additionally, leveraging HTTP compression and optimizing network requests can help minimize response times.
Lastly, monitoring and profiling your PHP code can provide valuable insights into performance bottlenecks. Utilize tools like Xdebug or New Relic to identify areas of your code that require optimization. By analyzing performance metrics and making data-driven optimizations, you can continuously improve the efficiency of your PHP code.
To summarize, improving the performance of your PHP code requires a comprehensive understanding of various factors. By optimizing variables and data structures, optimizing database queries, implementing caching mechanisms, optimizing code execution, and monitoring performance, you can enhance the speed and efficiency of your PHP applications. Stay tuned for the next section where we will dive deeper into optimizing variables and data structures in PHP.
Identifying Bottlenecks in Your Code
Identifying bottlenecks in your PHP code is crucial for improving the performance of your applications. When it comes to enhancing the efficiency and responsiveness of your code, understanding the factors that impact PHP performance is key. One important aspect to consider is the efficient use of variables and data structures. Avoid unnecessary variables and arrays that consume memory and processing power. Additionally, choose the appropriate data types to optimize performance.
Another area to focus on is database optimization. Writing efficient SQL queries and properly indexing your database tables can significantly improve data retrieval speed. Regular analysis and optimization of your database can lead to substantial performance gains.
Caching is also an effective technique for enhancing PHP performance. By temporarily storing frequently accessed data in memory or disk-based caches, you can reduce the time spent on expensive computations or database queries. Implementing caching mechanisms such as opcode caching or utilizing tools like Redis or Memcached can greatly enhance the speed of your PHP code.
Optimizing code execution by minimizing I/O operations and reducing network latency can further improve performance. Consider using asynchronous programming techniques to handle time-consuming tasks without blocking the main execution flow. Additionally, leverage HTTP compression and optimize network requests to minimize response times.
Monitoring and profiling your PHP code is essential for identifying performance bottlenecks. Utilize tools like Xdebug or New Relic to analyze performance metrics and make data-driven optimizations. By continuously improving the efficiency of your PHP code, you can enhance the speed and responsiveness of your applications.
In the next section, we will delve deeper into optimizing variables and data structures in PHP, providing you with practical techniques to further improve the performance of your code.
Optimizing Database Queries
Optimizing database queries is crucial for improving the performance of your PHP code. Inefficient queries can lead to slow data retrieval and increased load times, negatively impacting the user experience. Here are some key strategies to consider when optimizing database queries in PHP:
1. Use Indexes: Indexes are a powerful tool for enhancing query performance. By properly indexing your database tables, you can significantly speed up query execution. Analyze your queries and identify the columns frequently used in WHERE or JOIN clauses, then create indexes on those columns.
2. Query Optimization: Review your queries and ensure they are optimized for performance. Avoid using unnecessary JOINs and WHERE conditions. Use EXPLAIN or similar tools to analyze query execution plans and identify any potential bottlenecks. Optimize your queries based on the results to improve efficiency.
3. Limit Results: When fetching data from the database, only retrieve the necessary columns and limit the number of rows returned. This can reduce the amount of data transferred and improve query performance. Use techniques like pagination or LIMIT clauses to fetch only the required data.
4. Data Caching: Implement caching mechanisms to store frequently accessed data in memory. This can greatly reduce the need for repetitive database queries, improving performance. Consider using tools like Memcached or Redis to cache query results or objects.
5. Prepared Statements: Utilize prepared statements instead of dynamically building queries. Prepared statements not only provide protection against SQL injection attacks but also improve query execution by allowing the database to optimize query plans and reuse execution plans for similar queries.
6. Database Indexing: Regularly analyze and optimize your database indexes. Unused or redundant indexes can impact performance negatively. Remove any unnecessary indexes and ensure that the remaining ones are properly designed to support your query workload.
By implementing these strategies, you can significantly improve the performance of your PHP code by optimizing your database queries. Enhancing query execution, utilizing indexes, and implementing caching mechanisms will result in faster data retrieval and reduced load times, ultimately improving the overall user experience.
Using Caching Techniques
To improve the performance of your PHP code, it is essential to focus on using caching techniques. Caching can greatly enhance the speed and efficiency of your applications by storing frequently accessed data in memory or disk-based caches. By temporarily storing this data, you can reduce the time spent on expensive computations or database queries, ultimately providing a smoother user experience.
There are several caching mechanisms you can implement to optimize the performance of your PHP code. One popular option is opcode caching, which stores precompiled script bytecode in memory. This eliminates the need for PHP to repeatedly parse and compile the code, resulting in faster execution times.
Another caching technique is the use of tools like Redis or Memcached. These distributed caching systems allow you to store data in memory, providing high-speed access to frequently accessed information. By utilizing these tools, you can reduce the load on your database and improve the overall performance of your PHP code.
In addition to caching frequently accessed data, you can also take advantage of HTTP caching. This involves configuring your server to cache static assets, such as images or CSS files, and setting appropriate cache control headers. By caching these assets, you can minimize the number of requests made to the server, reducing network latency and improving the loading speed of your web pages.
When implementing caching techniques, it is important to consider cache invalidation. You need to ensure that the cache is updated when data changes to avoid serving stale information to users. This can be achieved through various strategies, such as setting expiration times for cached data or using cache tags to invalidate specific sets of data.
By incorporating caching techniques into your PHP code, you can significantly improve its performance. Whether through opcode caching, distributed caching systems, or HTTP caching, caching allows you to reduce the time spent on expensive operations and improve the overall responsiveness of your applications. So, take the time to evaluate your code and implement the appropriate caching mechanisms to optimize the performance of your PHP code.
Minimizing HTTP Requests
The performance of your PHP code plays a vital role in the overall user experience of your web applications. As your codebase grows, it’s essential to focus on improvements that can enhance its efficiency and responsiveness. One key area to target is minimizing HTTP requests.
Reducing the number of HTTP requests can significantly optimize the performance of your PHP code. Each request carries overhead in terms of time and resources, so minimizing them can lead to faster load times for your web pages. Here are a few strategies to help you achieve this:
1. Combine and Minify Files: By consolidating multiple CSS and JavaScript files into a single file and minifying them, you can reduce the number of HTTP requests required to fetch these assets. Minification removes unnecessary spaces, comments, and line breaks, resulting in smaller file sizes and faster downloads.
2. Use CSS Sprites: CSS sprites involve combining multiple images into a single image file and utilizing CSS background positioning to display specific sections of the image where needed. This technique reduces the number of image requests required, enhancing performance.
3. Implement Lazy Loading: Lazy loading involves loading images or other content only when it becomes visible to the user, rather than loading everything upfront. This approach reduces the initial number of HTTP requests and improves page load times, especially for long-scrolling pages or pages with abundant media content.
4. Utilize Caching: Implementing caching mechanisms can significantly reduce the number of HTTP requests by storing static assets in the browser cache. Setting appropriate cache headers and leveraging browser caching can ensure that returning users have a faster experience without requesting the same resources repeatedly.
5. Optimize AJAX Requests: If your PHP code relies on AJAX requests, make sure to minimize them by consolidating multiple related requests into one. Additionally, consider implementing data caching on the server-side to avoid unnecessary round trips to the server for the same data.
By implementing these strategies to minimize HTTP requests, you can greatly improve the performance of your PHP code. Remember to regularly analyze and evaluate your application to identify areas where request reduction can be applied. Enhancing the efficiency of your code will ultimately result in faster load times, improved user experience, and better overall performance.
Reducing CPU and Memory Usage
To improve the performance of your PHP code, reducing CPU and memory usage is essential. By optimizing your code to be more efficient, you can enhance the overall speed and responsiveness of your applications. Here are some key strategies to help you achieve this:
1. Limit Variable Usage: Avoid excessive use of variables and arrays, as they consume memory and processing power. Use only the necessary variables and consider the appropriate data types to optimize performance.
2. Optimize Loops and Functions: Analyze your loops and functions to identify any unnecessary iterations or redundant operations. Streamline your code by eliminating unnecessary steps and improving algorithm efficiency.
3. Use Memory Management Techniques: Implement techniques like object recycling and resource cleanup to efficiently utilize memory. Be mindful of creating and destroying objects or resources unnecessarily, as it can impact performance.
4. Optimize Database Queries: Inefficient database queries can put a strain on CPU and memory. Ensure that your queries are properly optimized by using indexes, limiting results, and implementing caching mechanisms to reduce the need for repetitive queries.
5. Utilize Opcode Caching: Implement opcode caching to store precompiled script bytecode in memory. This eliminates the need for repeated parsing and compilation of PHP scripts, resulting in faster execution times and reduced CPU usage.
6. Implement Caching: Use caching techniques to store frequently accessed data in memory or disk-based caches. This reduces the need for repeated computations or database queries, resulting in improved performance and reduced CPU usage.
7. Profile and Monitor Performance: Regularly monitor and profile your PHP code to identify performance bottlenecks. Utilize tools like Xdebug or New Relic to analyze performance metrics and make data-driven optimizations.
By implementing these strategies, you can significantly improve the performance of your PHP code by reducing CPU and memory usage. Optimizing variables and data structures, optimizing database queries, implementing caching mechanisms, and monitoring performance will result in faster and more efficient applications. Take the time to analyze your code and identify areas for improvement, and continuously optimize your codebase for optimal performance.
Implementing Code Profiling
Implementing Code Profiling
Improving the performance of your PHP code requires a thorough understanding of its execution and bottlenecks. Code profiling is a valuable technique that allows you to identify areas of your code that consume excessive resources or contribute to slow execution. By profiling your code, you can pinpoint specific functions or sections that need optimization, resulting in significant performance improvements.
There are several tools and techniques available for PHP code profiling. One popular tool is Xdebug, which provides a range of profiling features such as function-level profiling, memory usage tracking, and code coverage analysis. By enabling profiling in Xdebug and executing your code, you can generate profiling reports that highlight the time and resources consumed by each function.
Another option is to use a performance monitoring tool like New Relic. This tool provides comprehensive insights into your PHP application’s performance, including detailed transaction traces, database query analysis, and resource utilization. By monitoring your application in real-time or analyzing historical data, you can identify performance bottlenecks and make targeted optimizations.
When profiling your code, pay attention to functions or sections that have high execution times or consume excessive memory. Look for opportunities to optimize loops, reduce unnecessary function calls, or improve algorithm efficiency. Consider caching frequently accessed data or optimizing database queries to minimize the time spent on expensive operations.
It’s important to note that code profiling should be performed in a controlled environment and on representative use cases. Profiling production environments can introduce additional overhead and may not accurately reflect typical usage scenarios. Therefore, it’s recommended to profile your code on staging or development environments to get reliable results.
In conclusion, implementing code profiling is a valuable technique for improving the performance of your PHP code. By using tools like Xdebug or New Relic, you can identify performance bottlenecks and make targeted optimizations. Analyze the profiling reports, optimize resource-intensive functions, and consider caching or database query optimizations. By continuously profiling and optimizing your code, you can enhance the overall performance and responsiveness of your PHP applications.
Utilizing Opcode Caching
To improve the performance of your PHP code, it is crucial to focus on optimizing various aspects of your application. By implementing effective techniques and best practices, you can enhance the efficiency and responsiveness of your code. One essential area to consider is opcode caching. Opcode caching involves storing precompiled script bytecode in memory, eliminating the need for repetitive parsing and compilation of PHP scripts. This results in faster execution times and reduced CPU usage. Tools like Redis or Memcached can also be utilized for caching frequently accessed data, reducing the time spent on expensive computations or database queries. By leveraging caching mechanisms, you can significantly enhance the speed and efficiency of your PHP code. Additionally, minimizing HTTP requests is another effective strategy for improving performance. By combining and minifying files, using CSS sprites, implementing lazy loading, and optimizing AJAX requests, you can reduce the number of requests made to the server, resulting in faster load times. Furthermore, focusing on reducing CPU and memory usage is crucial for optimal performance. By optimizing variables and data structures, optimizing database queries, and implementing caching, you can minimize resource consumption and enhance the overall efficiency of your code. Lastly, implementing code profiling is a valuable technique for identifying performance bottlenecks and making targeted optimizations. Tools like Xdebug and New Relic provide insights into the execution of your PHP code, helping you pinpoint areas that require improvement. By continuously analyzing and optimizing your code, you can ensure that your PHP applications deliver a superior user experience with optimal performance.
Conclusion
Improving the performance of your PHP code is crucial for optimizing the speed and efficiency of your web applications. By focusing on various aspects of your code, you can enhance its overall performance and provide a better user experience. One key area to consider is opcode caching. By storing precompiled script bytecode in memory, opcode caching eliminates the need for repetitive parsing and compilation of PHP scripts, resulting in faster execution times and reduced CPU usage. Additionally, utilizing caching mechanisms such as Redis or Memcached can further enhance performance by storing frequently accessed data in memory, reducing the time spent on expensive computations or database queries.
Another strategy for improving performance is minimizing HTTP requests. By consolidating and minifying files, using CSS sprites, implementing lazy loading, and optimizing AJAX requests, you can reduce the number of requests made to the server and achieve faster load times. Additionally, focusing on reducing CPU and memory usage is essential. Optimizing variables and data structures, optimizing database queries, and implementing caching techniques can help minimize resource consumption and enhance the efficiency of your code.
Furthermore, implementing code profiling can provide valuable insights into performance bottlenecks. By analyzing the execution of your PHP code using tools like Xdebug or New Relic, you can identify areas that require optimization and make targeted improvements. Continuous monitoring and optimization of your code will ensure that your PHP applications deliver a superior user experience with optimal performance.
In conclusion, improving the performance of your PHP code requires a comprehensive approach. By leveraging opcode caching, minimizing HTTP requests, reducing CPU and memory usage, and implementing code profiling, you can optimize the speed, efficiency, and overall performance of your PHP applications. By continuously analyzing and optimizing your codebase, you can provide a seamless user experience and stay ahead in today’s fast-paced digital landscape.