Generated by Contentify AI
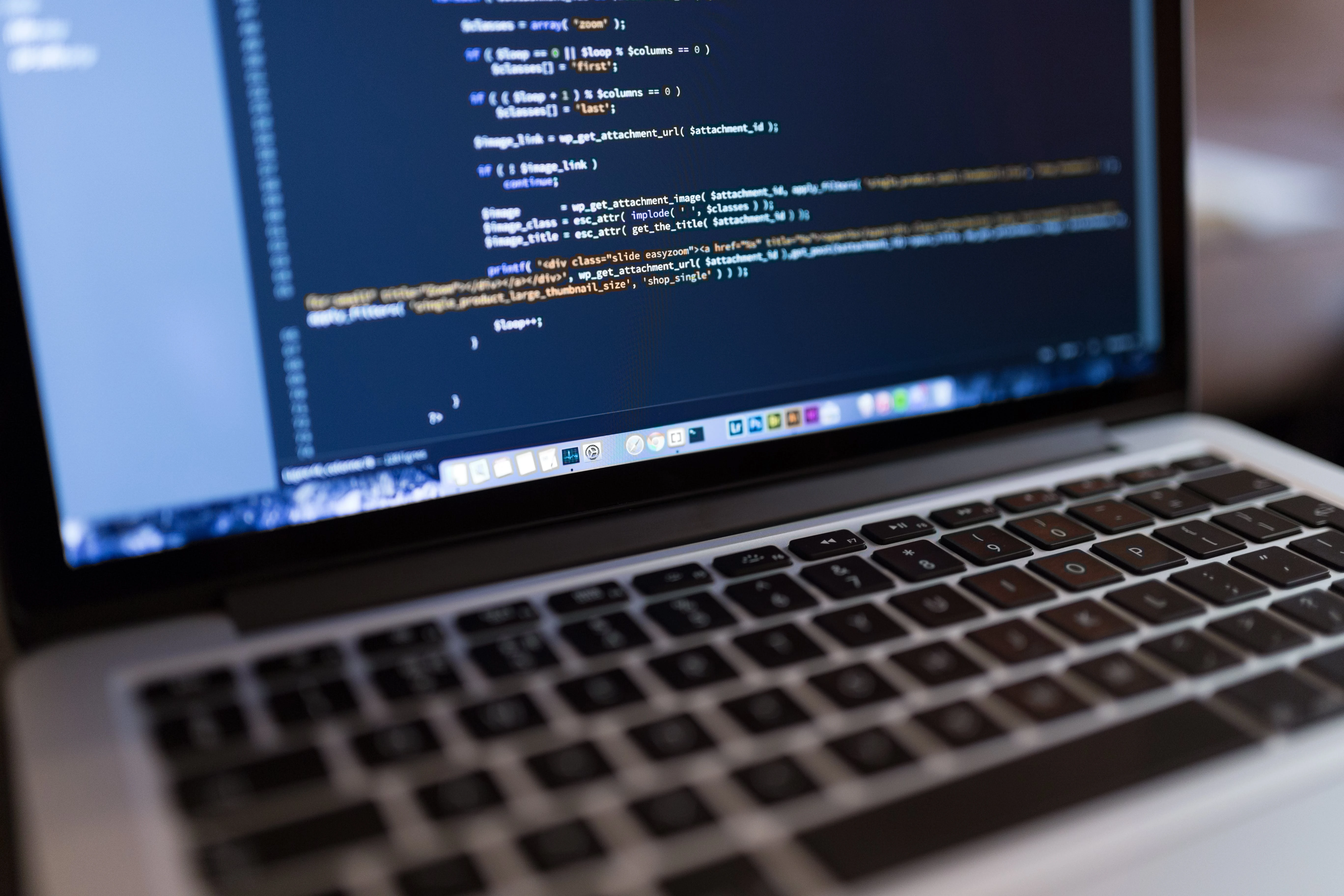
Introduction
When it comes to developing web applications using PHP, ensuring the security of your code is essential. With the increasing number of cyber threats and attacks, it is crucial to take the necessary steps to protect your PHP code from potential vulnerabilities. This article will provide you with valuable tips on how to secure your PHP code, reducing the risk of unauthorized access and potential security breaches. By following these best practices, you can enhance the security of your PHP applications, safeguarding sensitive data and ensuring the overall integrity of your code.
Understanding the Importance of Code Security
Developing secure PHP code is crucial in order to protect your web applications from potential vulnerabilities and cyber attacks. By understanding the importance of code security, you can take proactive steps to safeguard your PHP code and ensure the integrity of your applications. Implementing industry best practices and following security guidelines can significantly reduce the risk of unauthorized access and data breaches. In this article, we will explore various strategies and techniques for securing your PHP code, allowing you to develop robust and resilient web applications.
Common Vulnerabilities in PHP Code
Developing web applications using PHP is a common practice. However, it is important to ensure the security of your PHP code to protect against potential vulnerabilities. By understanding common vulnerabilities, you can take necessary measures to secure your code. One common vulnerability is SQL injection, where malicious code is injected into SQL queries. To prevent this, you should use prepared statements and parameterized queries. Another vulnerability is cross-site scripting (XSS), where attackers inject malicious scripts into web pages. To mitigate this, you should sanitize user input and use output encoding. Additionally, insecure file uploads can lead to arbitrary code execution. To address this, validate and sanitize files before storing or executing them. Lastly, inadequate user authentication and session management can result in unauthorized access. To enhance security, use strong password hashing algorithms and implement secure session management techniques. By understanding and addressing these common vulnerabilities, you can significantly improve the security of your PHP code.
Implementing Secure Coding Practices
Implementing Secure Coding Practices
Securing your PHP code is essential to protect your web applications from potential vulnerabilities and cyber attacks. By implementing secure coding practices, you can significantly reduce the risk of unauthorized access and data breaches. Here are some important steps to consider when securing your PHP code:
1. Input Validation: Validate all user input to prevent malicious data from being processed or executed. Use filtering and validation functions to ensure that only expected data types and formats are accepted.
2. Parameterized Queries: Use prepared statements and parameterized queries to prevent SQL injection attacks. This technique ensures that user input is treated as data rather than executable code.
3. Output Encoding: Sanitize and encode all user-generated content before displaying it on web pages. This helps prevent cross-site scripting (XSS) attacks by neutralizing any potential malicious scripts.
4. Secure Session Management: Implement secure session management techniques, such as using unique session IDs, setting session timeouts, and regenerating session IDs after login. This helps protect against session hijacking and session fixation attacks.
5. Password Hashing: Properly hash and salt user passwords using strong algorithms like bcrypt or Argon2. Avoid using weak hashing functions like MD5 or SHA1, as they are susceptible to brute-force attacks.
6. File Upload Validation: Validate and sanitize all file uploads to prevent arbitrary file execution and unauthorized access. Restrict file types, check for file size limits, and scan uploaded files for malware or malicious code.
7. Error Handling: Avoid displaying detailed error messages to users, as they may contain sensitive information that attackers can exploit. Instead, log errors securely and display generic error messages to users.
8. Regularly Update PHP and Libraries: Keep your PHP version and any third-party libraries up to date with the latest security patches. This helps protect against known vulnerabilities and exploits.
9. Secure Configuration: Ensure that your PHP server and application are configured securely. Disable unnecessary features, limit file permissions, and use secure communication protocols like HTTPS.
10. Regular Code Audits and Penetration Testing: Conduct regular code audits and penetration testing to identify and address security vulnerabilities in your PHP code. This helps uncover any weaknesses that may have been overlooked during development.
By following these secure coding practices, you can enhance the security of your PHP code and protect your web applications from potential threats. Remember that security is an ongoing process, and it’s important to stay updated with the latest security best practices and emerging threats.
Using Frameworks and Libraries to Enhance Security
Developing secure PHP code is of utmost importance to protect your web applications from potential vulnerabilities and cyber attacks. By implementing secure coding practices, you can significantly reduce the risk of unauthorized access and data breaches. One effective way to enhance the security of your PHP code is by leveraging frameworks and libraries. These tools provide pre-built functionalities and security measures that can strengthen your code’s defenses.
Frameworks like Laravel, Symfony, and CodeIgniter offer built-in security features such as input validation, data sanitization, and protection against common web vulnerabilities. By using these frameworks, you can ensure that your PHP code follows secure coding practices without having to reinvent the wheel. Additionally, these frameworks often have active communities, providing regular updates and patches to address security issues promptly.
Similarly, libraries like PHPMailer and SwiftMailer help secure email functionality by preventing email header injections and ensuring secure transmission of sensitive information. These libraries have undergone rigorous security testing and are trusted by developers worldwide.
By incorporating these frameworks and libraries into your PHP projects, you can benefit from their battle-tested security measures and save valuable development time. However, it is crucial to keep these tools updated to avoid using outdated versions that may have known vulnerabilities. Regularly check for updates and patch your dependencies to maintain a secure codebase.
In conclusion, securing your PHP code is vital for protecting your web applications from potential threats. By utilizing frameworks and libraries, you can enhance the security of your code without compromising functionality. Ensure that you stay up-to-date with the latest versions and security patches for these tools to maintain a robust and resilient codebase.
Regular Code Auditing and Testing
Regular Code Auditing and Testing
To ensure the security of your PHP code, regular code auditing and testing are essential. It helps identify potential vulnerabilities and ensures that your code is free from flaws. By following these steps, you can enhance the security of your PHP applications:
1. Code Review: Conduct thorough code reviews to identify any potential security issues or vulnerabilities. This involves analyzing the code line by line to detect any coding mistakes or insecure practices.
2. Penetration Testing: Perform penetration testing to simulate real-world attacks and identify any weak points in your application. This testing helps uncover vulnerabilities that may not be apparent during regular code review.
3. Security Scanning: Utilize automated security scanning tools to scan your code for known vulnerabilities and weaknesses. These tools can help identify common issues, such as SQL injection or cross-site scripting, and provide recommendations for fixing them.
4. Input Validation: Validate all user input to prevent malicious data from being processed or executed. This involves using filtering and validation functions to ensure that only expected data types and formats are accepted.
5. Error Handling: Implement proper error handling techniques to avoid exposing sensitive information. Avoid displaying detailed error messages to users, as they may provide valuable information to attackers.
6. Regular Updates: Keep your PHP version and any third-party libraries up to date with the latest security patches. This helps protect against known vulnerabilities and exploits.
7. Secure Configuration: Ensure that your PHP server and application are configured securely. Disable unnecessary features, limit file permissions, and use secure communication protocols like HTTPS.
By regularly auditing and testing your PHP code, you can identify and address any security vulnerabilities or weaknesses. This proactive approach helps reduce the risk of unauthorized access and data breaches, ensuring the overall security of your PHP applications.
Protecting Sensitive Data
Protecting Sensitive Data in PHP Code
When developing web applications using PHP, it is crucial to prioritize the security of sensitive data. By implementing proper measures, you can safeguard important information from unauthorized access. Here are some key steps to protect sensitive data in your PHP code:
1. Encryption: Encrypt sensitive data before storing it in your database or transmitting it over the network. Use strong encryption algorithms like AES or RSA to ensure that the data remains secure even if it falls into the wrong hands.
2. Secure File Storage: When storing sensitive data in files, ensure that they are stored outside of the web root directory. Restrict access permissions to these files to prevent unauthorized access. It is also recommended to encrypt the files before storing them.
3. Secure Database Configuration: Configure your database access properly by using strong passwords and limiting access privileges. Avoid using default database credentials and regularly update them to prevent unauthorized access.
4. Use Parameterized Queries: Utilize prepared statements or parameterized queries when interacting with databases. This helps prevent SQL injection attacks and ensures that user input is treated as data rather than executable code.
5. Password Hashing: Hash passwords using strong and up-to-date hashing algorithms like bcrypt or Argon2. Salting the passwords adds an extra layer of security and makes it harder for attackers to crack them.
6. Reducing Data Exposure: Minimize the amount of sensitive data stored in your application. Only collect and store the data that is necessary for your application’s functionality. This reduces the potential impact of a data breach.
7. Regularly Update and Patch: Keep your PHP version, frameworks, and libraries up to date with the latest security patches. This helps protect against known vulnerabilities and exploits.
8. Secure Session Management: Implement secure session management techniques, such as using unique session IDs, setting session timeouts, and regenerating session IDs after login. This prevents session hijacking and session fixation attacks.
By following these best practices, you can enhance the security of your PHP code and protect sensitive data from potential threats. Prioritizing the protection of sensitive information will ensure the integrity and confidentiality of your web applications.
Securing File and Directory Permissions
Securing the File and Directory Permissions of your PHP Code
Ensuring the security of your PHP code goes beyond implementing secure coding practices. It also involves securing the file and directory permissions of your codebase. Properly managing file and directory permissions is crucial to prevent unauthorized access and protect sensitive data. Here are some essential tips on how to secure your PHP code by managing file and directory permissions:
1. Restrict File Permissions: Set appropriate file permissions to limit access to your PHP files. Only grant read, write, and execute permissions to the necessary users or groups. Avoid giving unnecessary permissions that could potentially expose your code to unauthorized modifications or executions.
2. Secure Directory Permissions: Similarly, secure directories by setting proper permissions. Ensure that sensitive directories, such as those containing configuration files or user uploads, are only accessible to the required users or groups. Limiting access to these directories prevents unauthorized modifications or data breaches.
3. Avoid World-Writable Permissions: Avoid granting world-writable permissions to files or directories whenever possible. World-writable permissions allow any user on the server to modify or delete files, increasing the risk of unauthorized access or malicious actions. Instead, set permissions that only allow necessary users or groups to make changes.
4. Implement Strong Ownership: Assign appropriate ownership to your PHP files and directories. Assigning ownership to the least privileged user or group necessary for operation helps minimize the risk of unauthorized modifications. Additionally, ensure that ownership is regularly audited and updated as needed.
5. Regularly Monitor Permissions: Regularly monitor file and directory permissions to identify any unauthorized changes. Implementing a monitoring system that alerts you of any unexpected permission modifications can help you detect and respond to potential security breaches promptly.
By following these guidelines for managing file and directory permissions, you can strengthen the security of your PHP code. Remember, securing your PHP code is an ongoing process that requires vigilance and regular updates. Stay proactive in maintaining the integrity and confidentiality of your PHP applications by properly managing file and directory permissions.
Implementing Access Controls and Input Validation
Implementing Access Controls and Input Validation
Securing your PHP code is crucial to protect your web applications from potential vulnerabilities and cyber attacks. Two essential practices to enhance the security of your PHP code are implementing access controls and input validation.
Access controls play a vital role in ensuring that only authorized users can access certain functionalities or resources within your application. By implementing role-based access control (RBAC) or attribute-based access control (ABAC), you can define and enforce fine-grained permissions for different user roles. This helps prevent unauthorized access and restricts potential attackers from exploiting sensitive functionalities.
Input validation is another critical aspect of securing your PHP code. By validating and sanitizing all user input, you can prevent malicious data from being processed or executed. Utilize filtering and validation functions to ensure that only expected data types and formats are accepted. This helps mitigate common vulnerabilities such as SQL injection and cross-site scripting (XSS).
To implement proper input validation, consider using built-in PHP functions like filter_var() and filter_input(). These functions allow you to validate user input against specific filters, such as validating email addresses or checking if a value is an integer.
Additionally, it is crucial to sanitize user input before displaying it on web pages. This prevents potential XSS attacks by neutralizing any malicious scripts that may be injected. Utilize output encoding functions like htmlspecialchars() to encode special characters and HTML entities when displaying user-generated content.
By implementing access controls and input validation, you can significantly enhance the security of your PHP code. These practices help prevent unauthorized access, protect against common web vulnerabilities, and ensure the integrity of your applications. Regularly review and update your access control policies and input validation mechanisms to adapt to evolving security threats and stay ahead of potential attacks.
Remember, securing your PHP code is an ongoing process that requires diligence and adherence to best practices. By implementing access controls and input validation, you can fortify your PHP applications against malicious actors and safeguard your sensitive data.
Conclusion
One of the most important considerations when developing web applications using PHP is ensuring the security of your code. With the increasing prevalence of cyber threats and attacks, it is crucial to take proactive steps to protect your PHP code from vulnerabilities. By following best practices and implementing security measures, you can significantly enhance the security of your PHP applications. In this section, we will explore various techniques and strategies to secure your PHP code effectively.
One key aspect of securing your PHP code is implementing access controls. By defining and enforcing access permissions for different user roles, you can prevent unauthorized access to sensitive functionalities and resources within your application. Role-based access control (RBAC) or attribute-based access control (ABAC) are effective methods for managing access controls and ensuring that only authorized users can perform specific actions. By implementing these access control mechanisms, you can significantly reduce the risk of unauthorized access and potential security breaches.
Another important practice for securing your PHP code is input validation. Validating and sanitizing user input is crucial to prevent malicious data from being processed or executed. By utilizing filtering and validation functions, you can ensure that only expected data types and formats are accepted. This helps mitigate common vulnerabilities such as SQL injection and cross-site scripting (XSS). Additionally, it is crucial to sanitize user input before displaying it on web pages to prevent potential XSS attacks. By implementing proper input validation and output encoding, you can protect your PHP code from potential security threats.
In addition to access controls and input validation, there are several other techniques you can employ to secure your PHP code. These include implementing secure session management techniques, using strong password hashing algorithms, securing file and directory permissions, and regularly updating your PHP version and libraries with the latest security patches. Regular code auditing, penetration testing, and monitoring are also crucial to identify and address any security vulnerabilities or weaknesses in your PHP code.
Securing your PHP code is an ongoing process that requires vigilance and adherence to best practices. By following the techniques and strategies outlined in this section, you can enhance the security of your PHP applications and protect them from potential vulnerabilities and cyber attacks. By prioritizing the security of your PHP code, you can safeguard sensitive data, ensure the integrity of your applications, and provide a secure user experience.