Generated by Contentify AI
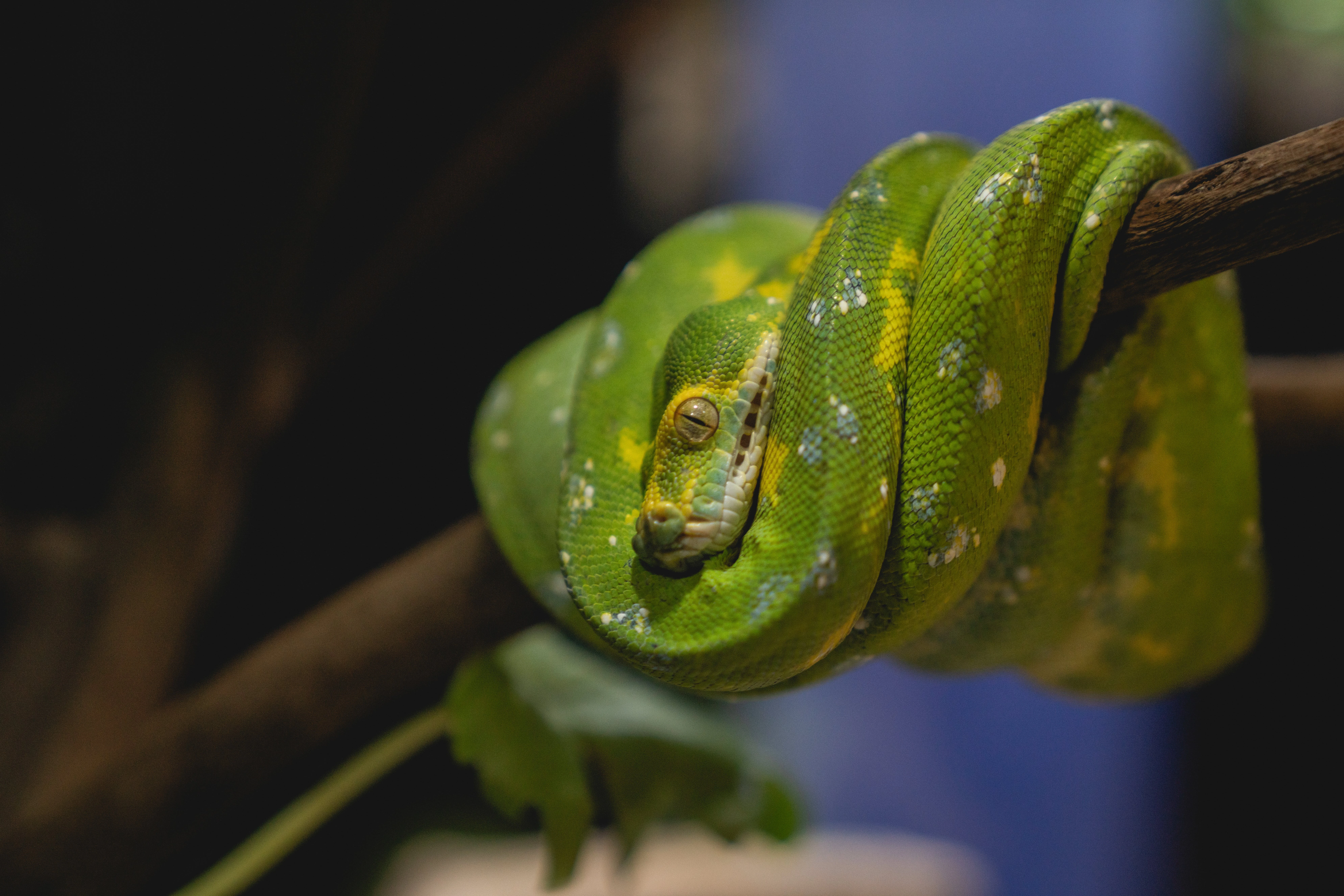
Introduction
Python is a versatile and powerful programming language that offers many features to simplify and optimize code. One such feature is the ability to use for loops, which allow us to iterate over a sequence of elements and perform actions on each one. In this section, we will explore the power of Python for loops and how they can enhance our programming capabilities. By understanding the syntax and functionality of for loops, we can unlock new possibilities for solving problems and efficiently processing data. So, let’s dive into the world of Python for loops and discover their potential.
What is a Python for loop?
Python for loops are a fundamental tool in programming that allow us to iterate over a sequence of elements. They provide a concise and efficient way to perform repetitive tasks, making them a powerful feature in Python. With a for loop, we can easily process a list, tuple, string, or any other iterable object in a systematic manner. By combining a for loop with conditional statements, we can add even more flexibility and control to our code. Whether we need to calculate the sum of numbers, filter specific elements, or perform complex calculations, Python for loops provide us with the necessary structure and control flow. By exploring the power of Python for loops, we can streamline our code, improve efficiency, and solve problems more effectively.
How to use a for loop in Python
Python for loops are a powerful tool for iterating over a sequence of elements in Python. They provide a concise and efficient way to perform repetitive tasks, making them an essential feature for any programmer. By using a for loop, we can easily process lists, tuples, strings, or any other iterable object in a systematic manner. Additionally, by combining a for loop with conditional statements, we can add flexibility and control to our code. The power of Python for loops lies in their ability to streamline code, improve efficiency, and solve problems effectively. Whether we need to calculate sums, filter elements, or perform complex calculations, for loops provide the necessary structure and control flow. Exploring the power of Python for loops allows us to unlock new possibilities and enhance our programming capabilities.
Examples of for loops in Python
Python‘s for loops are a powerful feature that allows programmers to iterate over a sequence of elements efficiently. By exploring the various ways to use for loops, we can harness their power and unlock new possibilities in our code.
One example of using a for loop in Python is to iterate over a list of numbers and calculate their sum. By using a for loop, we can iterate over each element in the list, add it to a running total, and finally return the sum. This eliminates the need for writing repetitive code and simplifies the process of summing a list of numbers.
Another example of utilizing Python’s for loops is to iterate over a string and perform a specific action on each character. For instance, we can iterate over a string and convert each character to uppercase or lowercase, depending on our needs. This allows us to manipulate strings easily and perform tasks such as text cleaning or data transformation.
Furthermore, for loops can be used to iterate over a range of numbers and perform complex calculations. By specifying the start and end points of the range, we can execute a series of operations on each number in the range. This can be particularly useful when dealing with mathematical computations or generating sequences of numbers.
In conclusion, exploring the power of Python for loops opens up a world of possibilities for programmers. Whether it’s iterating over lists, strings, or ranges, for loops provide an efficient and concise way to process data. By understanding and utilizing the functionality of for loops, we can enhance our programming capabilities and solve problems more effectively.
Tips and tricks for using for loops effectively
Python for loops are a powerful tool for iterating over a sequence of elements in Python. By exploring their capabilities, we can enhance our programming skills and solve problems more effectively. Here are some tips and tricks to use for loops effectively:
1. Understand the syntax: Familiarize yourself with the syntax of a for loop in Python. It typically consists of the keyword ‘for’, followed by a variable name, the keyword ‘in’, and an iterable object.
2. Choose the right iterable: Determine the appropriate iterable object to loop over. It could be a list, tuple, string, range, or any other iterable object. Each type of iterable has its unique characteristics and use cases.
3. Use conditional statements: Combine for loops with conditional statements to add flexibility to your code. By incorporating if-else statements within the loop, you can perform different actions based on certain conditions.
4. Leverage built-in functions: Take advantage of built-in functions such as ‘len()’, ‘range()’, and ‘enumerate()’ to simplify your for loops. These functions provide useful features to iterate over sequences and manipulate data.
5. Avoid unnecessary iterations: Optimize your code by avoiding unnecessary iterations. If possible, break out of the loop or use ‘continue’ to skip iterations that are not required.
6. Consider list comprehensions: In some cases, list comprehensions can provide a more concise and efficient way to achieve the same results as a for loop. Explore this alternative syntax and see if it fits your specific use case.
7. Test and debug your code: Always test your for loops with different inputs and verify that they produce the desired output. Use print statements or debugging tools to identify any errors or unexpected behavior.
By following these tips and tricks, you can harness the power of Python for loops and write more efficient and effective code. Keep exploring and experimenting with for loops to unlock their full potential in your programming journey.
Advanced concepts and techniques with for loops
Python for loops offer advanced concepts and techniques that allow programmers to harness their power and enhance their programming capabilities. By exploring the various ways to utilize for loops in Python, developers can streamline their code, improve efficiency, and solve problems more effectively.
One of the key advantages of Python for loops is their ability to iterate over a sequence of elements, such as lists, tuples, or strings. By using a for loop, programmers can easily process each element in the sequence, perform actions on them, and achieve the desired result. This eliminates the need for writing repetitive code and simplifies complex tasks.
Furthermore, Python for loops can be combined with conditional statements, such as if-else statements, to add flexibility and control to the code. By incorporating conditional logic within a for loop, programmers can execute different actions based on specific conditions. This allows for more dynamic and adaptable code.
In addition to iterating over sequences, Python for loops can also be used to iterate over a range of numbers. This is particularly useful for performing mathematical calculations or generating sequences of numbers. By specifying the start and end points of the range, programmers can efficiently execute a series of operations on each number within the range.
To optimize the use of Python for loops, programmers can consider using built-in functions like len(), range(), or enumerate(). These functions provide useful features to iterate over sequences, manipulate data, and simplify the code.
It’s important to note that unnecessary iterations should be avoided to optimize the code. Programmers can use techniques like breaking out of the loop or using the continue statement to skip iterations that are not required. By doing so, the code can be more efficient and performant.
Lastly, testing and debugging the code that uses for loops is crucial. Programmers should thoroughly test their for loops with different inputs to ensure they produce the desired output. This can be done by using print statements or debugging tools to identify any errors or unexpected behavior.
In conclusion, exploring the power of Python for loops allows programmers to unlock new possibilities and enhance their programming capabilities. By understanding the syntax, leveraging conditional statements, and using built-in functions effectively, programmers can streamline their code, improve efficiency, and solve problems more effectively. Continued exploration and experimentation with for loops will undoubtedly lead to further mastery of this powerful programming feature.
Common mistakes to avoid with for loops
Common mistakes to avoid with for loops
When exploring the power of Python for loops, it is important to be aware of common mistakes that programmers may make. By understanding these mistakes and how to avoid them, we can ensure that our for loops are effective and efficient. Here are some common mistakes to watch out for when using for loops in Python:
1. Modifying the iterable: One common mistake is modifying the iterable object while iterating over it. This can lead to unexpected behavior and errors. To avoid this, it is recommended to create a copy of the iterable or use a different approach, such as iterating over a copy of the list.
2. Overusing nested loops: Nested loops can quickly become complex and decrease the performance of our code. It is important to avoid unnecessary nesting and consider alternative approaches, such as using list comprehensions or built-in functions like map() or filter().
3. Forgetting to update the loop variable: It is crucial to update the loop variable properly within the loop body. Missing or incorrect updates can result in infinite loops or incorrect results. Always make sure to update the loop variable appropriately to ensure the desired behavior.
4. Not using break or continue statements effectively: The break statement allows us to exit the loop prematurely, while the continue statement allows us to skip the current iteration. Not using these statements effectively can lead to inefficiencies in our code. Be sure to use break and continue statements appropriately to optimize the execution of for loops.
5. Ignoring error handling: When performing operations within a for loop, it is important to consider error handling. Ignoring error handling can result in unexpected exceptions that may disrupt the flow of our program. Always handle exceptions appropriately within the loop to ensure smooth execution.
6. Using for loops for large data sets: For loops may not be the most efficient approach when dealing with large data sets. In such cases, it is recommended to explore alternative techniques like using vectorized operations or employing libraries specifically designed for efficient data manipulation, such as NumPy or pandas.
By avoiding these common mistakes, we can harness the full power of Python for loops and ensure that our code is efficient, error-free, and capable of handling various scenarios. With a clear understanding of these pitfalls, we can confidently navigate the world of for loops and leverage them to their full potential.
Conclusion
In the world of Python programming, one essential tool that developers rely on is the for loop. By exploring the power of Python for loops, codecamp.org/news/how-to-think-like-a-programmer-lessons-in-problem-solving-d1d8bf1de7d2/” target=”_blank”>programmers can streamline their code and solve problems more effectively. With a concise syntax and the ability to iterate over sequences, for loops offer a versatile solution for processing lists, tuples, strings, and other iterable objects. Combining for loops with conditional statements adds flexibility and control to the code, enabling programmers to execute different actions based on specific conditions. Furthermore, Python for loops can be used to perform mathematical calculations, generate sequences of numbers, and manipulate data efficiently. While using for loops, it is important to avoid common mistakes such as modifying the iterable, overusing nested loops, and neglecting error handling. By following best practices, programmers can harness the power of Python for loops and enhance their programming capabilities.