Generated by Contentify AI
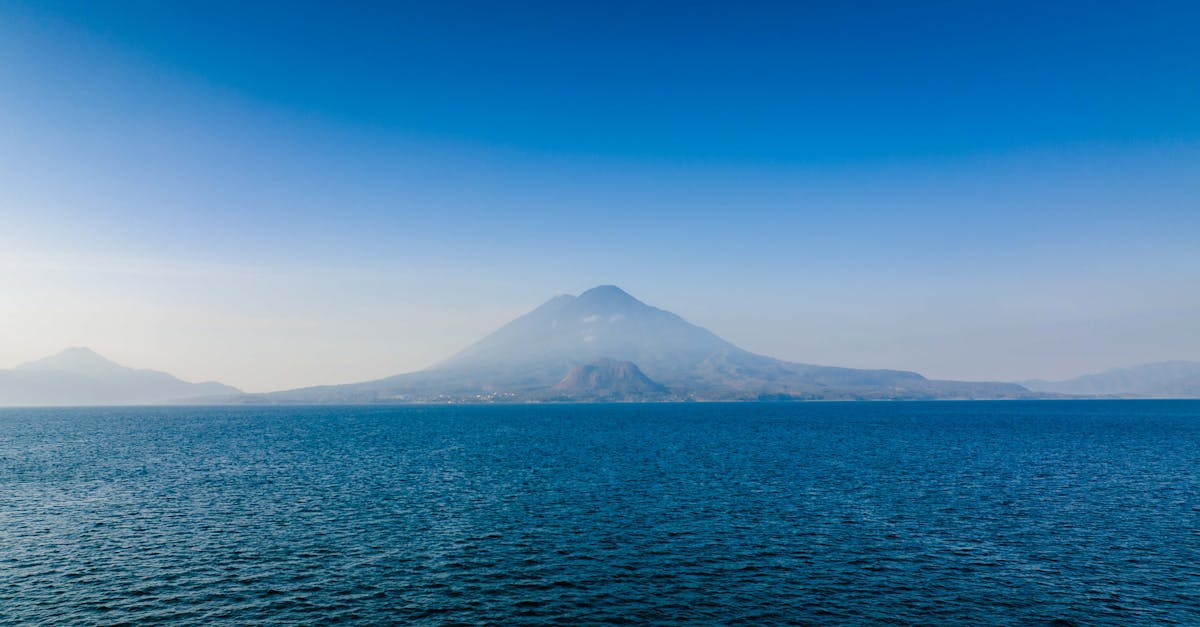
Creating and initializing an ArrayList is a common task for Java developers. An ArrayList is a dynamic array that can grow or shrink in size based on the elements added or removed. This makes it a popular choice for storing and manipulating data in Java programs.
To create an ArrayList, you first need to import the java.util.ArrayList class. This can be done by adding the following line of code at the top of your Java file:
“`java
import java.util.ArrayList;
“`
Once you have imported the class, you can create an ArrayList using the following syntax:
“`java
ArrayList myArrayList = new ArrayList();
“`
In this example, we have created a new ArrayList object called myArrayList that can store Strings. You can replace String with any other data type to create an ArrayList of that data type.
Initializing an ArrayList is the process of adding elements to it. There are several ways to initialize an ArrayList in Java. One way is to add elements one by one using the add() method. For example:
“`java
ArrayList myArrayList = new ArrayList();
myArrayList.add(“apple”);
myArrayList.add(“banana”);
myArrayList.add(“orange”);
“`
In this example, we have created an ArrayList of Strings and added three elements to it: “apple”, “banana”, and “orange”.
Another way to initialize an ArrayList is to pass a collection of elements to the constructor. For example:
“`java
ArrayList myArrayList = new ArrayList(
Arrays.asList(“apple”, “banana”, “orange”));
“`
In this example, we have created an ArrayList of Strings and initialized it with three elements using the Arrays.asList() method.
In conclusion, creating and initializing an ArrayList is a fundamental task for Java developers. By following the steps outlined above, you can easily create and manipulate dynamic arrays of any data type.