Generated by Contentify AI
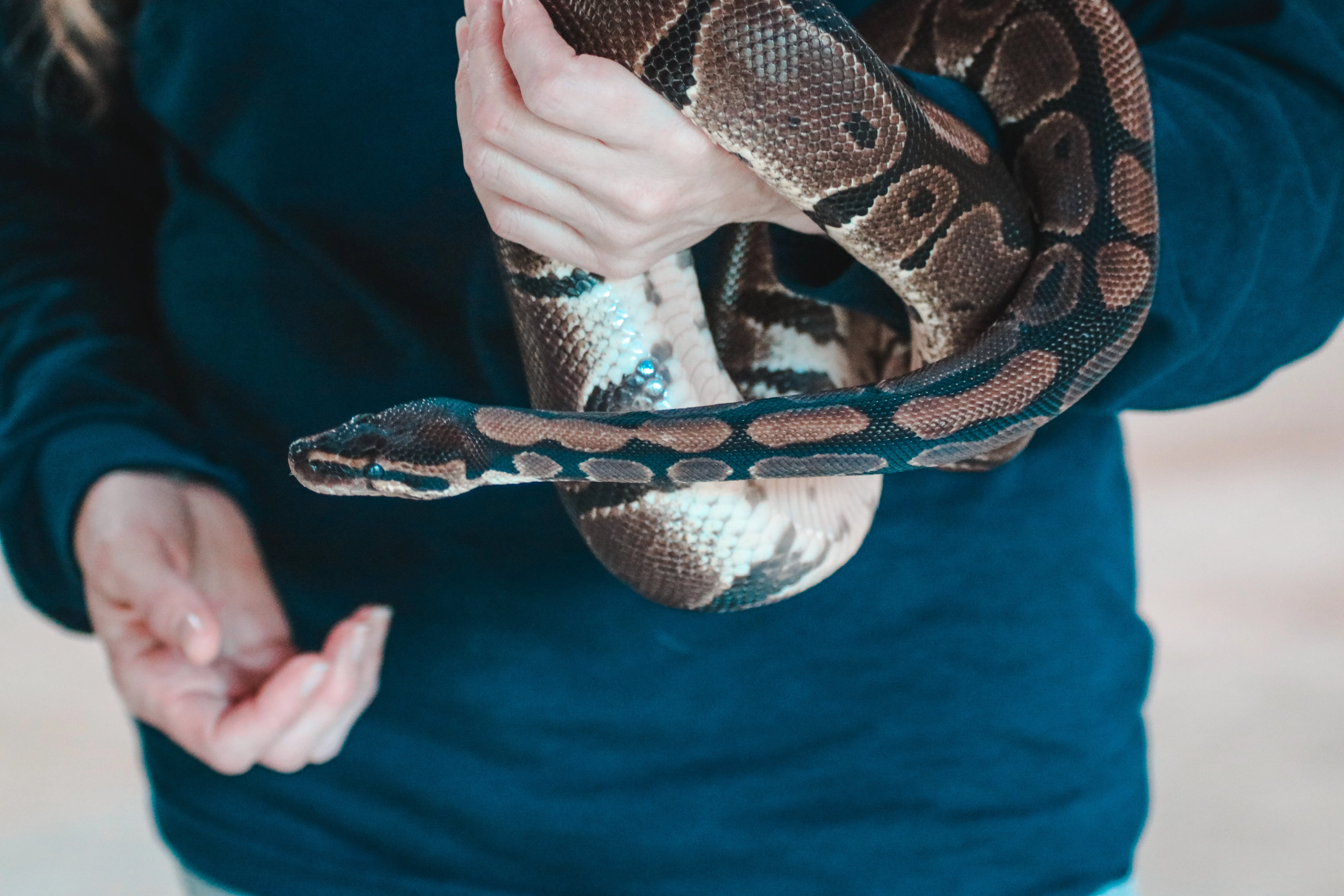
Introduction
When using Python for loops, it is important to be aware of common mistakes that can occur. These mistakes can lead to errors in the code or inefficient execution. By understanding and avoiding these mistakes, you can ensure that your for loops are functioning correctly and efficiently. In this section, we will discuss some of the common mistakes to avoid when using Python for loops.
Neglecting Indentation
The proper indentations are crucial when working with Python for loops. Neglecting proper indentation is a common mistake that can lead to syntax errors and unexpected results. In Python, indentation is used to define the scope of a loop or a block of code. Forgetting to indent the code within a for loop can cause the loop to be skipped entirely or result in incorrect iteration. On the other hand, indenting code that should not be part of the loop will cause it to repeat unnecessarily. To avoid these mistakes, always ensure that the code within a for loop is indented correctly and that any code outside of the loop is not indented. Proper indentation is essential for the correct execution and functionality of your Python for loops.
Not Using Meaningful Variable Names
Not Using Meaningful Variable Names
One common mistake to avoid when using Python for loops is not using meaningful variable names. It can be tempting to use generic or single-letter variable names, especially when writing short loops. However, this practice can make your code difficult to understand and maintain.
Using descriptive variable names is essential for readability and to convey the purpose of the loop. It helps both you and other developers to easily understand the logic and intentions behind your code. Meaningful variable names make your code self-explanatory and reduce the chances of introducing bugs or errors.
Instead of using generic names like ‘i’ or ‘x’, choose names that accurately represent the data being iterated over. For example, if you’re looping through a list of names, use a variable name like ‘name’ or ‘person’ to make the code more readable.
By using meaningful variable names, you enhance the clarity and maintainability of your code, making it easier to debug and modify in the future. So, remember to always use descriptive names when using Python for loops, and avoid the common mistake of using vague or uninformative variable names.
Misusing the Range Function
Misusing the Range Function
One of the common mistakes to avoid when using Python for loops is misusing the range function. The range function is often used to generate a sequence of numbers that can be iterated over in a for loop. However, it is important to understand how the range function works to avoid errors and unexpected behavior.
A common mistake is not understanding that the range function generates numbers up to, but not including, the specified end value. For example, if you want to iterate over a sequence of numbers from 1 to 5, you would use range(1, 6) instead of range(1, 5). This ensures that the loop includes the number 5 in the iteration.
Another mistake is not utilizing the start and step parameters of the range function. By default, the start parameter is 0 and the step parameter is 1. However, you can specify different values to customize the sequence of numbers generated by the range function. For example, range(1, 10, 2) will generate a sequence of odd numbers from 1 to 9.
Misusing the range function can lead to off-by-one errors or incorrect iteration. It is important to carefully consider the start, end, and step values when using the range function in a for loop.
In conclusion, avoiding common mistakes when using Python for loops is crucial for the correct functionality and efficiency of your code. Misusing the range function can lead to errors and unexpected results. By understanding how the range function works and using it correctly, you can ensure that your for loops operate as intended.
Forgetting to Use Break or Continue Statements
Neglecting to Use Break or Continue Statements
When working with Python for loops, it is essential to remember to utilize break and continue statements. These statements provide control over the execution of the loop and help avoid common mistakes. By neglecting to use break or continue, you may encounter issues such as unnecessary iterations or incorrect termination of the loop.
Forgetting to include a break statement can result in the loop continuing to execute even after a certain condition has been met. This can lead to inefficient code execution and potential bugs. On the other hand, omitting a continue statement can cause the loop to proceed with the next iteration without executing the remaining code within the loop body. This can result in incorrect data processing or skipped computations.
To avoid these mistakes, it is crucial to include break and continue statements at the appropriate locations within the loop body. The break statement allows you to exit the loop prematurely when a specific condition is met, while the continue statement enables you to skip the remaining code and proceed to the next iteration. Proper usage of these statements ensures efficient execution and accurate results within your Python for loops.
In conclusion, when using Python for loops, it is important to avoid the common mistakes of neglecting to use break or continue statements. By incorporating these statements correctly, you can control the flow of your loop and prevent unnecessary iterations or incorrect termination.
Modifying the Iterated List
Modifying the Iterated List
A common mistake to avoid when working with Python for loops is modifying the iterated list. It is important to understand that when you iterate over a list in a for loop, you should not modify the list itself. Modifying the list while iterating can lead to unpredictable behavior and errors in your code.
Instead, create a separate list or variable to store any modifications you need to make. Iterate over the original list and perform the necessary operations on the separate list or variable. This ensures that the original list remains unchanged and the iteration proceeds smoothly.
Modifying the iterated list not only introduces errors but also results in inefficient code execution. The length of the list changes dynamically as you modify it, impacting the loop’s iteration and potentially skipping elements.
To avoid this mistake, separate the modification process from the iteration process. By doing so, you can maintain the integrity of the original list and ensure accurate results in your Python for loops.
In conclusion, modifying the iterated list is a common mistake to avoid when using Python for loops. By keeping the original list intact and performing modifications separately, you can prevent errors and ensure efficient and accurate execution of your code.
Confusing the Syntax of Nested Loops
Confusing the Syntax of Nested Loops
Nested loops, where one loop is placed inside another, are a powerful tool in Python programming. However, they can also be a source of confusion, especially when it comes to the syntax. One common mistake to avoid when using Python for loops is getting confused with the syntax of nested loops.
The key to avoiding this mistake is understanding that each loop has its own indentation level. The inner loop should be indented further than the outer loop to indicate that it is nested inside. Failure to correctly indent the inner loop will result in a syntax error.
Additionally, it is important to keep track of the number of iterations and the order in which the nested loops are executed. In some cases, the inner loop may need to iterate completely before the outer loop can move to the next iteration. Understanding and managing the flow of nested loops is essential to achieving the desired results.
To avoid this common mistake, it is recommended to double-check the indentation levels of nested loops and carefully plan the order of execution. By doing so, you can ensure that your nested loops are syntactically correct and produce the intended outcomes.
Using an Infinite Loop
Nested loops, where one loop is placed inside another, can be a powerful tool in Python programming. However, they can also be a source of confusion if not properly understood. One common mistake to avoid when using Python for loops is getting confused with the syntax of nested loops.
The key to avoiding this mistake is understanding that each loop has its own indentation level. The inner loop should be indented further than the outer loop to indicate that it is nested inside. Failure to correctly indent the inner loop will result in a syntax error.
Additionally, it is important to keep track of the number of iterations and the order in which the nested loops are executed. In some cases, the inner loop may need to iterate completely before the outer loop can move to the next iteration. Understanding and managing the flow of nested loops is essential to achieving the desired results.
To avoid this common mistake, it is recommended to double-check the indentation levels of nested loops and carefully plan the order of execution. By doing so, you can ensure that your nested loops are syntactically correct and produce the intended outcomes.
Not Using List Comprehension
When working with Python for loops, it is important to be aware of common mistakes that can hinder your code’s functionality and efficiency. One such mistake is neglecting to use list comprehension. List comprehension is a powerful feature in Python that allows you to create lists in a concise and efficient manner. By using list comprehension, you can avoid the need for explicit for loops and simplify your code. Neglecting to utilize list comprehension can result in longer and more complex code, making it harder to read and maintain. It is important to familiarize yourself with the syntax and capabilities of list comprehension to take advantage of its benefits. By avoiding this common mistake and using list comprehension when appropriate, you can simplify your code and improve its readability.
Conclusion
When working with Python for loops, it is important to be aware of common mistakes that can hinder your code’s functionality and efficiency. One such mistake is neglecting proper indentation. Neglecting to indent the code within a for loop can lead to syntax errors and unexpected results. Another mistake is using generic or vague variable names, which can make your code difficult to understand and maintain. Misusing the range function, such as forgetting that it generates numbers up to, but not including the specified end value, can result in off-by-one errors. Not utilizing the break or continue statements can lead to unnecessary iterations or incorrect termination of the loop. Modifying the iterated list while iterating can cause unpredictable behavior and errors in your code. Lastly, confusing the syntax of nested loops can result in syntax errors. By avoiding these common mistakes and following best practices, you can ensure that your Python for loops are functioning correctly and efficiently.