Generated by Contentify AI
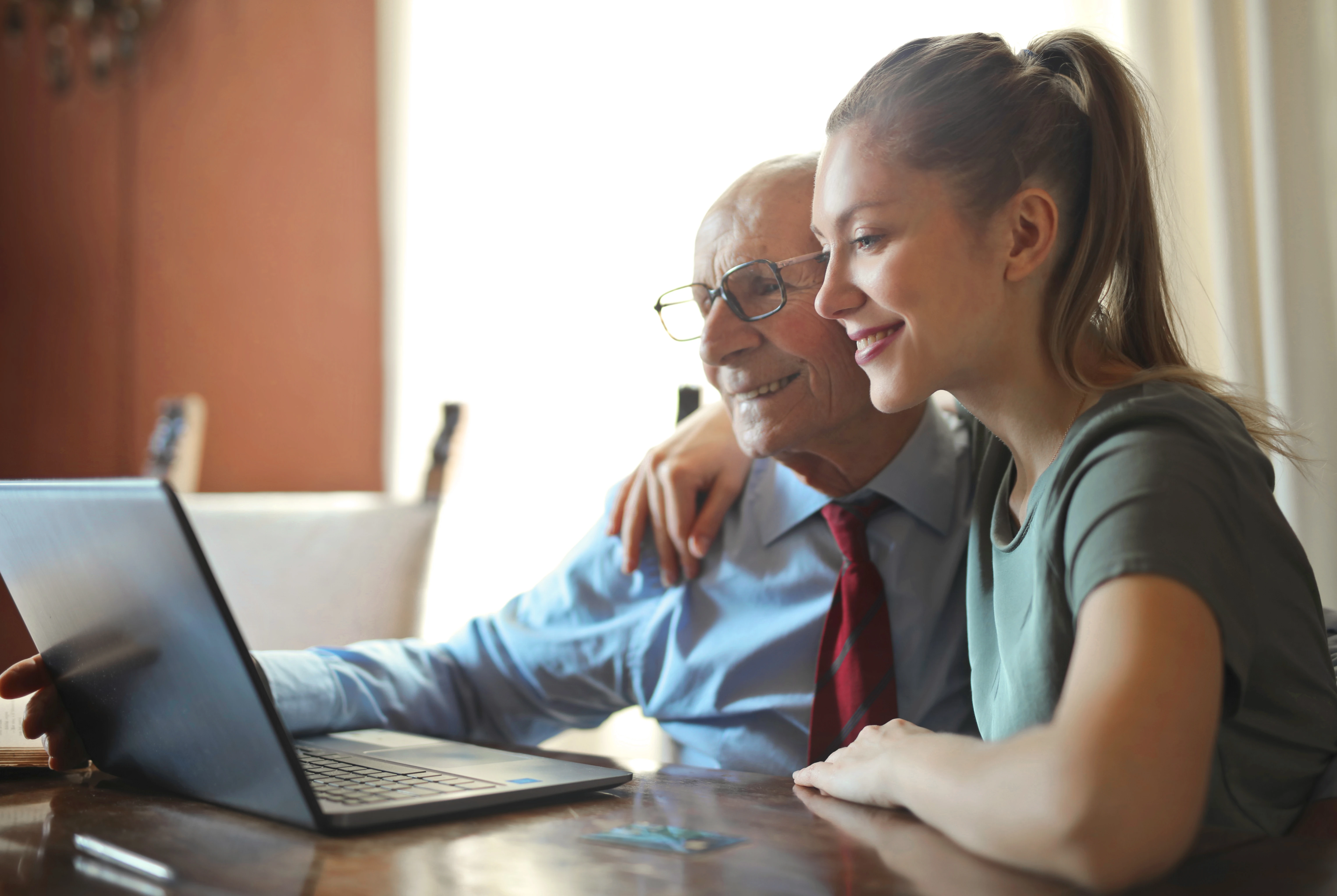
Introduction
jQuery is a powerful JavaScript library that simplifies the process of building CRUD applications. CRUD stands for Create, Read, Update, and Delete, which are the basic operations performed on data in a database. With jQuery, developers can easily manipulate the DOM, handle user interactions, and perform AJAX requests, making it an ideal choice for building interactive and responsive web applications.
When building CRUD applications with jQuery, developers can take advantage of its extensive set of features and plugins. jQuery provides a wide range of functions for selecting and manipulating HTML elements, handling events, and making asynchronous requests to the server. This makes it easier to create forms for data input, display data in tables or lists, and update or delete records without having to refresh the entire page.
One of the key benefits of using jQuery for CRUD applications is its ability to handle data validation and form submission. jQuery’s validation plugin allows developers to easily define validation rules for form fields, ensuring that data is entered correctly before it is submitted to the server. This helps to maintain data integrity and improve the overall user experience.
Additionally, jQuery’s AJAX capabilities enable developers to perform asynchronous requests to the server, allowing for seamless data retrieval and manipulation without disrupting the user’s workflow. This means that users can view, edit, and delete data without experiencing page reloads or interruptions.
In summary, building CRUD applications with jQuery offers developers a streamlined approach to creating interactive and responsive web applications. With its extensive set of features and plugins, jQuery simplifies the process of handling user interactions, validating form data, and performing asynchronous requests. Whether you are a beginner or an experienced developer, jQuery can greatly enhance your ability to build powerful and efficient CRUD applications.
What is CRUD?
CRUD, which stands for Create, Read, Update, and Delete, is a fundamental concept in database management. It refers to the basic operations performed on data. When it comes to building CRUD applications, jQuery is a powerful tool that simplifies the process. With jQuery, developers can easily manipulate the DOM, handle user interactions, and perform AJAX requests.
One of the key benefits of using jQuery for CRUD applications is its ability to handle data validation and form submission. jQuery’s validation plugin allows developers to easily define validation rules for form fields, ensuring that data is entered correctly before it is submitted to the server. This helps to maintain data integrity and improve the overall user experience.
jQuery also provides extensive features and plugins for selecting and manipulating HTML elements, making it easier to create forms for data input, display data in tables or lists, and update or delete records without having to refresh the entire page.
Another advantage of using jQuery for CRUD applications is its AJAX capabilities. Developers can perform asynchronous requests to the server, allowing for seamless data retrieval and manipulation without disrupting the user’s workflow. This means that users can view, edit, and delete data without experiencing page reloads or interruptions.
In summary, building CRUD applications with jQuery offers developers a streamlined approach to creating interactive and responsive web applications. jQuery’s extensive features and plugins make it easier to handle user interactions, validate form data, and perform asynchronous requests. Whether you are a beginner or an experienced developer, jQuery can greatly enhance your ability to build powerful and efficient CRUD applications.
Overview of jQuery
jQuery is a widely used JavaScript library that simplifies the process of building CRUD applications. With its extensive set of features and plugins, it offers developers a streamlined approach to creating interactive and responsive web applications.
One of the key advantages of using jQuery for CRUD applications is its ability to handle data validation and form submission. By leveraging jQuery’s validation plugin, developers can easily define validation rules for form fields, ensuring that data is entered correctly before it is submitted to the server. This not only helps maintain data integrity but also enhances the overall user experience.
In addition to data validation, jQuery provides a wide range of functions for selecting and manipulating HTML elements. This makes it easy to create forms for data input, display data in tables or lists, and update or delete records without having to refresh the entire page.
Furthermore, jQuery’s AJAX capabilities enable developers to perform asynchronous requests to the server. This allows for seamless data retrieval and manipulation without disrupting the user’s workflow. Users can view, edit, and delete data without experiencing page reloads or interruptions, resulting in a more efficient and user-friendly application.
In conclusion, building CRUD applications with jQuery offers developers a powerful and efficient solution. Whether you are a beginner or an experienced developer, jQuery’s extensive features and plugins can greatly enhance your ability to create interactive and responsive web applications.
Setting up the Development Environment
Setting up the Development Environment
Before diving into building CRUD applications with jQuery, it is important to set up the development environment properly. This ensures a smooth and efficient development process. Here are some steps to follow:
1. Install jQuery: Begin by downloading the latest version of jQuery from the official website or using a package manager like npm or Bower. Include the jQuery library in your project by adding the script tag to your HTML file.
2. HTML Structure: Create the necessary HTML structure for your application. This includes setting up containers, forms, tables, or any other elements required to display and interact with data.
3. CSS Styling: Apply CSS styles to your HTML elements to create an appealing and user-friendly interface. Consider using a CSS framework like Bootstrap or Foundation to speed up the styling process.
4. Include AJAX Support: Since CRUD operations involve sending and receiving data from the server, it is important to include AJAX support in your application. jQuery’s AJAX capabilities make it easy to send requests to the server and handle the responses seamlessly.
5. Set up Server-side Code: Depending on your server-side technology, set up the necessary code to handle CRUD operations. This may involve creating RESTful APIs or server-side scripts to handle data manipulation.
6. Test and Debug: Test your application thoroughly to ensure that all CRUD operations are working correctly. Use browser developer tools to debug any issues that may arise during the development process.
By following these steps, you can set up a development environment that is ready to handle CRUD operations using jQuery. This will allow you to efficiently build powerful and interactive applications that can create, read, update, and delete data with ease.
Creating the Database Structure
Creating the Database Structure
When building CRUD applications with jQuery, one of the first steps is to create the database structure. This involves designing the tables, defining the relationships between them, and establishing the necessary fields and data types.
A well-designed database structure is crucial for efficient data management. It ensures that data is organized, stored, and retrieved accurately. When creating the database structure, consider the entities and attributes that will be part of your application. Identify the primary keys, foreign keys, and any constraints or indexes necessary for data integrity.
To create the database structure, you can use a database management system (DBMS) such as MySQL, PostgreSQL, or Microsoft SQL Server. These systems provide tools for creating tables, specifying column types, and establishing relationships between tables.
Once the database structure is defined, you can use jQuery to interact with the database and perform CRUD operations. jQuery provides functions for dynamically generating SQL queries, inserting new records, retrieving data, updating existing records, and deleting records.
When working with jQuery and databases, it is important to handle data validation and sanitization to prevent SQL injection attacks. jQuery’s validation plugin can be used to validate user input before it is inserted or updated in the database. Additionally, server-side validation should always be implemented to ensure data integrity and security.
In conclusion, creating the database structure is a crucial step in building CRUD applications with jQuery. It lays the foundation for efficient data management and allows for seamless interaction with the database. By following best practices and using jQuery’s features and plugins, developers can create robust and secure applications that effectively perform CRUD operations.
Designing the User Interface
Designing the User Interface
When it comes to building CRUD applications with jQuery, designing an intuitive and user-friendly interface is essential. A well-designed user interface enhances the user experience and makes it easier for users to interact with the application. Here are some key points to consider when designing the user interface for a CRUD application using jQuery.
1. Consistency: Maintain consistency throughout the application by using the same design elements, colors, and typography. This helps users navigate the application more easily and reduces confusion.
2. Clear Navigation: Ensure that the navigation is clear and easily accessible. Use menus, tabs, or breadcrumbs to help users understand where they are in the application and how to move between different sections.
3. Responsive Design: With the increasing use of mobile devices, it is important to design the user interface to be responsive. This means that the application should adapt to different screen sizes and resolutions, providing a consistent experience across devices.
4. Intuitive Forms: Use clear and descriptive labels for form fields and provide helpful instructions or placeholders when needed. Validate user input in real-time to provide immediate feedback and prevent errors.
5. Error Handling: Design the user interface to handle errors gracefully. Display meaningful error messages and provide suggestions for resolving the issue. Highlight the fields with errors to help users identify and correct their mistakes.
6. Interactive Elements: Make the interface more engaging by incorporating interactive elements such as buttons, checkboxes, and drop-down menus. Use jQuery’s event handling functions to add interactivity and enhance the user experience.
7. Feedback and Confirmation: Provide visual feedback when actions like record creation, update, or deletion are performed. Use alerts, notifications, or progress bars to let users know that their actions have been successfully processed.
8. Visual Hierarchy: Organize information in a hierarchical manner, highlighting the most important elements and making them stand out. Use appropriate font sizes, colors, and spacing to guide users’ attention to the key areas of the application.
By following these guidelines, you can design a user interface that is visually appealing, user-friendly, and efficient for building CRUD applications with jQuery. Remember to continuously gather user feedback and make improvements to ensure an optimal user experience.
Implementing the Create Functionality
Implementing the Create Functionality
One of the essential functions in a CRUD application is the ability to create new records. With jQuery, implementing the create functionality becomes a straightforward process.
To begin, you’ll need a form where users can input the data for the new record. You can create the form using HTML and style it using CSS to ensure it matches the overall design of your application. Use jQuery’s event handling functions to listen for the form submission event.
Once the user submits the form, you can use jQuery’s AJAX capabilities to send the data to the server. This can be done by making a POST request to the server-side script or RESTful API endpoint that handles the creation of new records.
Before submitting the data, it’s crucial to validate it to ensure it meets the required criteria. jQuery’s validation plugin can be used to define validation rules for the form fields. This helps in maintaining data integrity and prevents the submission of invalid data to the server.
On the server-side, you’ll need to handle the incoming data and perform the necessary operations to create a new record in the database. This may involve validating the data again and inserting it into the appropriate table.
Once the record is successfully created on the server, you can provide feedback to the user. This can be done by displaying a success message or redirecting the user to a page that confirms the successful creation of the record.
In summary, implementing the create functionality in a CRUD application with jQuery involves creating a form, validating the data, making an AJAX request to the server, handling the data on the server-side, and providing feedback to the user. By leveraging jQuery’s features and plugins, you can easily implement the create functionality and enable users to add new records to your application.
Implementing the Read Functionality
Implementing the Read Functionality
In building CRUD applications with jQuery, the implementation of the Read functionality is a crucial step. The Read functionality allows users to retrieve and view data from the database. With jQuery, this process becomes simple and efficient.
To implement the Read functionality, start by designing the user interface to display the data. This can be done using HTML and CSS, creating tables or lists to present the information in a structured format. jQuery’s extensive features and plugins can assist in selecting and manipulating HTML elements, making it easy to populate the interface with data from the server.
Next, use jQuery’s AJAX capabilities to send a request to the server and retrieve the data. This can be done by making a GET request to the server-side script or RESTful API endpoint that handles the Read operation. jQuery’s AJAX functions provide a seamless way to fetch data without refreshing the entire page.
Once the data is retrieved from the server, you can dynamically update the user interface using jQuery’s DOM manipulation methods. This allows for real-time updates to the displayed data without the need for page reloads. You can also incorporate features like search or pagination to enhance the user experience and make it easier for users to navigate through large datasets.
In summary, implementing the Read functionality in CRUD applications with jQuery involves designing the user interface, retrieving data from the server using AJAX, and dynamically updating the interface to display the information. By leveraging jQuery’s features and plugins, developers can create efficient and user-friendly applications that allow users to easily access and view data from the database.
Implementing the Update Functionality
Implementing the Update Functionality
The update functionality is a critical aspect of building CRUD applications with jQuery. It allows users to modify existing data in the database. With jQuery’s extensive set of features and plugins, implementing the update functionality becomes a straightforward process.
To begin, you’ll need a form or an interface where users can make changes to the data. This form can be pre-populated with the existing data to provide users with a reference. Use jQuery to listen for user interactions and capture the updated values.
Once the user submits the form or triggers the update action, use jQuery’s AJAX capabilities to send an update request to the server. This request can be made using the PATCH or PUT method to the server-side script or RESTful API endpoint responsible for updating records.
On the server-side, handle the update request and process the updated data. Validate the input to ensure data integrity and security. Update the corresponding record in the database with the new values provided by the user.
After updating the record on the server, provide feedback to the user to confirm the successful update. This can be done by displaying a success message or redirecting the user to a page that confirms the update.
In summary, implementing the update functionality in CRUD applications with jQuery involves designing a form or interface for users to make changes, using AJAX to send an update request to the server, handling the update on the server-side, and providing feedback to the user. By leveraging jQuery’s features and plugins, developers can easily implement the update functionality and enable users to modify existing data in the application.
Implementing the Delete Functionality
The Delete functionality is a crucial part of building CRUD applications with jQuery. It allows users to remove records from the database. Implementing the Delete functionality with jQuery is straightforward and efficient.
To implement the Delete functionality, you’ll need a mechanism for users to initiate the delete action. This can be done through a button or a link associated with each record. Use jQuery’s event handling functions to listen for the click event on the delete button or link.
Once the user initiates the delete action, use jQuery’s AJAX capabilities to send a delete request to the server. This request can be made using the DELETE method to the server-side script or RESTful API endpoint responsible for deleting records.
On the server-side, handle the delete request and identify the record to be deleted. Validate the request to ensure that the user has the necessary permissions to delete the record. Then, delete the record from the database.
After successfully deleting the record on the server, provide feedback to the user to confirm the successful deletion. This can be done by displaying a success message or updating the user interface to remove the deleted record.
In summary, implementing the Delete functionality in CRUD applications with jQuery involves providing users with a mechanism to initiate the delete action, using AJAX to send a delete request to the server, handling the delete on the server-side, and providing feedback to the user. By leveraging jQuery’s features and plugins, developers can easily implement the Delete functionality and allow users to remove records from the application.
Conclusion
The process of building CRUD applications with jQuery involves leveraging its extensive features and plugins. With jQuery, developers can easily manipulate the DOM, handle user interactions, and perform AJAX requests, making it an ideal choice for creating interactive and responsive web applications. One of the key benefits of using jQuery for CRUD applications is its ability to handle data validation and form submission. By utilizing jQuery’s validation plugin, developers can define validation rules for form fields, ensuring data integrity and improving the overall user experience. jQuery’s AJAX capabilities allow for seamless data retrieval and manipulation without disrupting the user’s workflow. This means users can view, edit, and delete data without experiencing page reloads or interruptions. Additionally, jQuery provides functions for selecting and manipulating HTML elements, making it easier to create forms for data input, display data in tables or lists, and update or delete records without having to refresh the entire page. Building CRUD applications with jQuery offers developers a streamlined approach to creating interactive and responsive web applications. Its extensive features and plugins simplify handling user interactions, validating form data, and performing asynchronous requests. jQuery enhances the ability to build powerful and efficient CRUD applications, regardless of the developer’s experience level.