Generated by Contentify AI
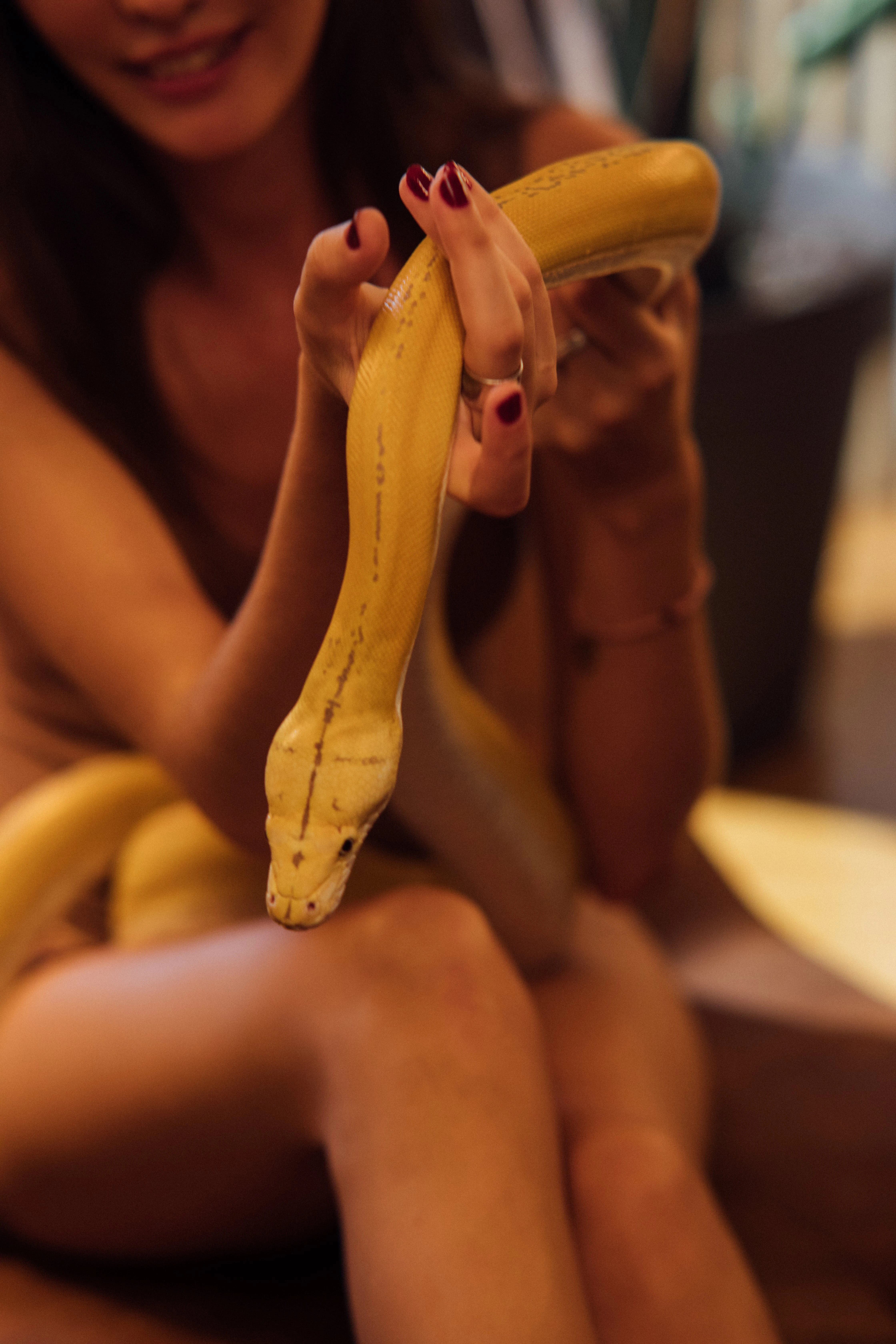
Introduction
Python for loops are a fundamental construct in programming, allowing us to iterate over a sequence of elements and perform operations on each one. However, there are advanced techniques that can enhance the functionality and efficiency of for loops in Python. These techniques can greatly improve the readability and performance of your code. In this section, we will explore some of these advanced techniques for Python for loops. By leveraging these techniques, you can write more concise and expressive code, handle complex iteration scenarios, and optimize the performance of your programs. So, let’s dive into the world of advanced techniques for Python for loops.
Understanding the Basics of Python For Loops
Python for loops are a powerful tool for iterating over sequences of elements. However, by exploring advanced techniques, we can take our for loops to the next level. One technique is using list comprehensions, which allows us to create new lists based on existing ones in a more concise and expressive way. Another technique is using the enumerate() function, which provides both the index and value of each element in the loop. This can be useful when we need to keep track of the position of each element. We can also use the zip() function to iterate over multiple lists simultaneously. This is especially handy when we need to perform operations on corresponding elements from different lists. Additionally, we can use the itertools module to generate iterable sequences that satisfy specific criteria. This can be useful when we need to iterate over combinations, permutations, or other types of sequences. Lastly, we can optimize the performance of our for loops by utilizing the timeit module. This allows us to measure the execution time of our code and identify any bottlenecks that need improvement. By mastering these advanced techniques, we can enhance the functionality and efficiency of our Python for loops.
Using Range() Function in Python For Loops
Python for loops are a fundamental construct in programming, allowing us to iterate over a sequence of elements and perform operations on each one. However, there are advanced techniques that can enhance the functionality and efficiency of for loops in Python.
One such technique is using the range() function. The range() function generates a sequence of numbers that can be used as an iterator in a for loop. It takes in three parameters: start, stop, and step. By default, start is 0 and step is 1.
Using range() in a for loop allows us to iterate over a specific range of numbers. This is particularly useful when we need to perform a repetitive task a certain number of times.
For example, if we want to print the numbers from 1 to 10, we can use the range() function in the following way:
“`python
for i in range(1, 11):
print(i)
“`
This will output:
“`
1
2
3
4
5
6
7
8
9
10
“`
By using range() in combination with a for loop, we can easily iterate over a specific range of numbers and perform operations on each one. This is just one of the advanced techniques that can be used to enhance the functionality and efficiency of Python for loops.
Iterating Through Lists and Strings Using Python For Loops
Python for loops are a fundamental construct in programming, allowing us to iterate over a sequence of elements and perform operations on each one. While the basic usage of for loops is well-known, there are advanced techniques that can enhance their functionality and efficiency. One such technique is iterating through lists and strings using Python for loops.
When it comes to iterating through lists, Python offers several advanced techniques. One such technique is list comprehension, which allows us to create new lists based on existing ones in a more concise and expressive way. With list comprehension, we can easily filter, transform, or manipulate elements within a list.
Another technique is using the enumerate() function, which provides both the index and value of each element in the loop. This can be especially useful when we need to keep track of the position of each element or when we want to access both the index and value simultaneously.
When it comes to iterating through strings, Python for loops can be used in a similar fashion. By treating a string as an iterable sequence of characters, we can iterate over each character and perform operations on them. This allows us to process and manipulate strings efficiently.
Overall, iterating through lists and strings using Python for loops is a powerful technique that allows us to process and manipulate data effectively. By leveraging advanced techniques like list comprehension and the enumerate() function, we can write more concise and expressive code. This not only improves the readability of our programs but also enhances their performance.
Using the Enumerate() Function in Python For Loops
Python for loops are a fundamental construct in programming, allowing us to iterate over a sequence of elements and perform operations on each one. While the basic usage of for loops is well-known, there are advanced techniques that can enhance their functionality and efficiency.
One such technique is using the enumerate() function. This function provides both the index and value of each element in the loop, allowing us to keep track of the position of each element. By leveraging the enumerate() function, we can easily access both the index and value simultaneously, making our code more expressive and readable.
Another advanced technique is using list comprehension. List comprehension allows us to create new lists based on existing ones in a more concise and expressive way. With list comprehension, we can filter, transform, or manipulate elements within a list, making our code more efficient and compact.
Additionally, we can use the zip() function to iterate over multiple lists simultaneously. This is particularly useful when we need to perform operations on corresponding elements from different lists. By leveraging the zip() function, we can process multiple lists in parallel, improving the efficiency of our code.
Furthermore, the itertools module provides advanced techniques for generating iterable sequences that satisfy specific criteria. This can be useful when we need to iterate over combinations, permutations, or other types of sequences. By utilizing the itertools module, we can handle complex iteration scenarios with ease.
Lastly, we can optimize the performance of our for loops by leveraging the timeit module. This module allows us to measure the execution time of our code and identify any bottlenecks that need improvement. By optimizing the performance of our for loops, we can ensure that our programs run efficiently.
In conclusion, by utilizing advanced techniques like the enumerate() function, list comprehension, zip() function, itertools module, and timeit module, we can enhance the functionality and efficiency of Python for loops. These techniques allow us to write more concise and expressive code, handle complex iteration scenarios, and optimize the performance of our programs.
Working with Nested Python For Loops
Python for loops are a fundamental construct in programming, allowing us to iterate over a sequence of elements and perform operations on each one. While the basic usage of for loops is well-known, there are advanced techniques that can enhance their functionality and efficiency.
One such advanced technique is working with nested for loops. In Python, we can nest for loops within each other to iterate over multiple sequences simultaneously. This can be useful when we have nested data structures or when we need to perform operations on combinations of elements from different sequences.
By using nested for loops, we can access and manipulate elements at different levels of nesting. For example, if we have a list of lists, we can use nested for loops to iterate through each sublist and each element within the sublist. This allows us to perform operations on individual elements or groups of elements within the nested structure.
Nested for loops can also be combined with other advanced techniques, such as list comprehension or the enumerate() function, to further enhance their functionality. For example, we can use list comprehension within nested for loops to create new lists based on specific conditions or transformations applied to the elements.
However, it is important to note that working with nested for loops can increase the complexity and potentially impact the performance of our code. Therefore, it is essential to carefully design and optimize nested for loops to ensure efficient execution.
In conclusion, working with nested for loops is an advanced technique that allows us to iterate over multiple sequences simultaneously and perform operations on elements at different levels of nesting. By leveraging this technique along with other advanced techniques, we can enhance the functionality and efficiency of our Python for loops.
Using the Break Statement in Python For Loops
Python for loops are a powerful tool for iterating over sequences of elements and performing operations on each one. While the basic usage of for loops is well-known, there are advanced techniques that can enhance their functionality and efficiency. One such technique is using the break statement.
The break statement allows us to prematurely exit a for loop based on a certain condition. This can be useful when we want to stop iterating once a specific condition is met and avoid unnecessary iterations. By strategically placing the break statement within our for loop, we can optimize the performance of our code and improve its efficiency.
For example, let’s say we have a list of numbers and we want to find the first occurrence of a specific number. We can use a for loop to iterate over the list and check each element. Once we find the desired number, we can use the break statement to exit the loop and stop further iterations.
“`
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
target = 5
for number in numbers:
if number == target:
print(“Number found!”)
break
“`
In this example, once the number 5 is found, the break statement is executed, and the loop is terminated. This saves unnecessary iterations and improves the efficiency of the code.
Using the break statement effectively requires careful consideration of the condition and placement within the for loop. It is important to ensure that the break statement is triggered at the appropriate moment to achieve the desired result.
In conclusion, the break statement is an advanced technique that can enhance the functionality and efficiency of Python for loops. By using the break statement strategically, we can optimize our code and improve its performance.
Using the Continue Statement in Python For Loops
Python for loops are a fundamental construct in programming, allowing us to iterate over a sequence of elements and perform operations on each one. While the basic usage of for loops is well-known, there are advanced techniques that can enhance their functionality and efficiency. One such technique is using the break statement. The break statement allows us to prematurely exit a for loop based on a certain condition. This can be useful when we want to stop iterating once a specific condition is met and avoid unnecessary iterations. Another advanced technique is using list comprehension, which allows us to create new lists based on existing ones in a more concise and expressive way. With list comprehension, we can easily filter, transform, or manipulate elements within a list. Additionally, we can use the enumerate() function to access both the index and value of each element in the loop. This can be especially useful when we need to keep track of the position of each element or when we want to access both the index and value simultaneously. Furthermore, by utilizing nested for loops, we can iterate over multiple sequences simultaneously and perform operations on elements at different levels of nesting. This can be useful when we have nested data structures or when we need to perform operations on combinations of elements from different sequences. Overall, these advanced techniques for Python for loops allow us to write more efficient and expressive code, handle complex iteration scenarios, and optimize the performance of our programs.
Using the Else Statement in Python For Loops
Python for loops are a fundamental construct in programming. While their basic usage is well-known, there are advanced techniques that can enhance their functionality and efficiency. One such technique is using the break statement. By strategically placing the break statement within a for loop, we can prematurely exit the loop based on a specific condition, avoiding unnecessary iterations and improving performance. Another advanced technique is list comprehension, which allows us to create new lists based on existing ones. This concise and expressive approach simplifies filtering, transforming, and manipulating elements within a list. Additionally, the enumerate() function provides both the index and value of each element in a loop, enabling us to keep track of position and access both index and value simultaneously. Lastly, nested for loops allow us to iterate over multiple sequences simultaneously, performing operations on elements at different levels of nesting. These advanced techniques for Python for loops empower us to write efficient and expressive code, handle complex iteration scenarios, and optimize program performance.
Conclusion
In the world of Python programming, for loops are a fundamental tool for iterating over sequences and performing operations on each element. However, there are advanced techniques that can take your for loops to the next level. These techniques allow you to write more concise and expressive code, handle complex iteration scenarios, and optimize the performance of your programs.
One such technique is using list comprehension, which allows you to create new lists based on existing ones in a more streamlined and expressive way. By leveraging list comprehension, you can easily filter, transform, or manipulate elements within a list, resulting in more efficient code.
Another advanced technique is the use of the enumerate() function, which provides both the index and value of each element in the loop. This is especially useful when you need to keep track of the position of each element or when you want to access both the index and value simultaneously. By utilizing the enumerate() function, you can enhance the readability and functionality of your for loops.
Nested for loops are yet another advanced technique that allows you to iterate over multiple sequences simultaneously. This is particularly useful when you have nested data structures or when you need to perform operations on combinations of elements from different sequences. By effectively utilizing nested for loops, you can efficiently process and manipulate complex data structures.
Additionally, utilizing control flow statements such as the break and continue statements can further enhance the functionality of your for loops. The break statement allows you to prematurely exit a loop based on a specific condition, while the continue statement allows you to skip the current iteration and proceed to the next one. These statements provide greater control and flexibility in your iteration process.
In conclusion, by incorporating advanced techniques such as list comprehension, the enumerate() function, nested for loops, and control flow statements, you can elevate the functionality and efficiency of your Python for loops. These techniques enable you to write more concise and expressive code, handle complex iteration scenarios, and optimize the performance of your programs.