Generated by Contentify AI
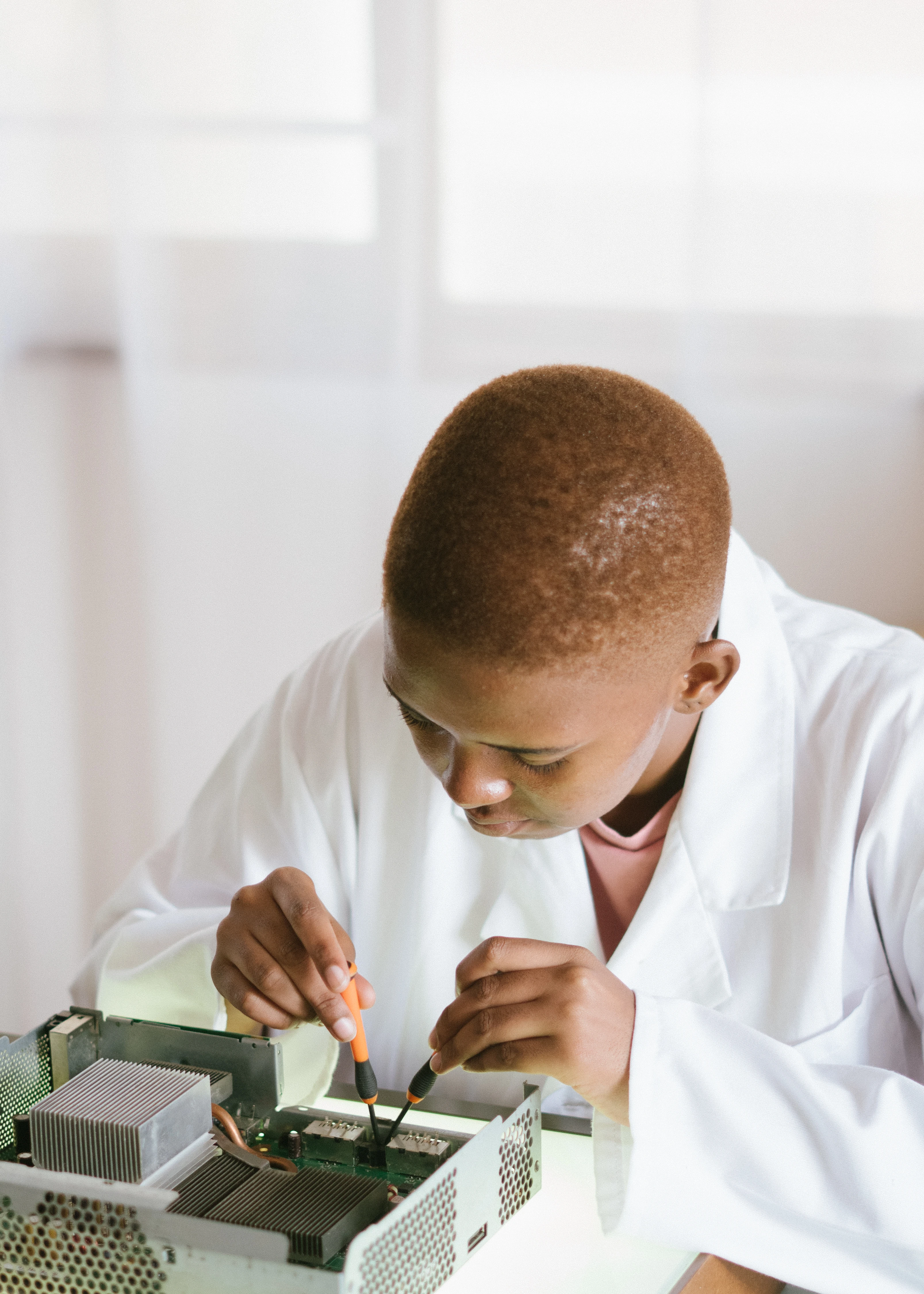
Introduction
jQuery is a popular JavaScript library that simplifies event handling on web pages. With its advanced event handling capabilities, jQuery allows developers to easily manage and respond to user interactions. By utilizing jQuery’s powerful event handling methods, developers can create dynamic and interactive web applications. In this section, we will explore the various advanced event handling techniques offered by jQuery, enabling you to enhance the user experience on your website. So, let’s dive into the world of advanced event handling with jQuery and discover how it can elevate your web development skills.
Understanding Event Handling in jQuery
Understanding Event Handling in jQuery
Event handling is a crucial aspect of web development, as it allows websites to respond dynamically to user interactions. jQuery provides a simplified and efficient way to handle events on web pages. With jQuery, developers can easily attach event handlers to HTML elements and define the actions to be performed when an event occurs. Additionally, jQuery offers a wide range of event methods, such as click, hover, submit, and keydown, allowing developers to listen for specific user actions. These methods can be combined with powerful selectors and event delegation techniques to handle events efficiently and improve performance. By understanding the principles of event handling in jQuery, developers can create interactive and responsive web applications that engage users and enhance their browsing experience.
Using Event Delegation
Event delegation is an advanced event handling technique in jQuery that allows developers to efficiently handle events on dynamically created or modified elements. Instead of attaching event handlers to each individual element, event delegation allows developers to attach a single event handler to a parent element that will be triggered for all matching child elements. This approach is particularly useful in scenarios where elements are added or removed from the DOM dynamically.
By using event delegation, developers can simplify their code and improve performance. When an event occurs, jQuery checks if the event target matches a specified selector. If it does, the event handler is executed. This way, even if new elements are added to the DOM after the event handler is attached, they will still trigger the event.
To implement event delegation in jQuery, developers can use the `on()` method. This method takes two arguments: the event type and the selector for the target elements. By delegating events to a parent element, developers can reduce the number of event handlers and improve the efficiency of their code.
In addition to event delegation, jQuery offers other advanced event handling features, such as event namespacing, event bubbling, and event stopping. These features provide further control and flexibility in managing events on web pages.
In conclusion, advanced event handling with jQuery, including event delegation, is a powerful tool for developers to efficiently handle events on dynamically created or modified elements. By using event delegation and other advanced techniques, developers can create interactive and responsive web applications that provide a seamless user experience.
Exploring Custom Events
Exploring Custom Events
jQuery‘s advanced event handling capabilities extend beyond the built-in event types. With jQuery, developers can create and utilize custom events to handle specific scenarios in their web applications. Custom events allow for greater flexibility and control in managing user interactions.
To create a custom event in jQuery, developers can use the `trigger()` method. This method triggers an event of a specified type on a selected element. By defining custom event types, developers can create unique interactions and actions.
Custom events can be used to enhance the user experience by creating custom animations, implementing complex workflows, or triggering specific functionality. For example, a web application may have a custom event for when a user completes a form submission. By triggering this custom event, developers can perform additional actions, such as displaying a success message or redirecting the user to a different page.
In addition to creating custom events, jQuery also provides methods for binding and unbinding custom event handlers. The `bind()` and `unbind()` methods allow developers to attach and detach event handlers to custom events, providing further control over event handling.
By exploring and utilizing custom events in jQuery, developers can create highly interactive and customized web applications. Custom events offer a level of flexibility and control that goes beyond the default event types, empowering developers to create unique and engaging user experiences.
In the next section, we will dive deeper into advanced event handling with jQuery by examining event namespacing and event delegation. These features further enhance the capabilities of event handling in jQuery, providing even more flexibility and control over user interactions.
Working with Event Object
Working with the Event Object
One of the key aspects of advanced event handling with jQuery is the ability to work with the event object. The event object contains valuable information about the event itself, such as the type of event, the target element, and any additional data associated with the event.
By accessing the event object, developers can extract and utilize this information to perform specific actions. For example, they can retrieve the value of an input field when a key is pressed or prevent the default action of a button click event.
To access the event object in jQuery, developers can pass it as a parameter to the event handler function. By doing so, they gain access to a range of properties and methods that provide detailed information about the event.
For instance, the `event.target` property allows developers to identify the specific element that triggered the event. This is especially useful when handling events on multiple elements with a single event handler.
Furthermore, the `event.preventDefault()` method can be used to prevent the default action associated with an event. This is commonly used to override the default behavior of an anchor tag or a form submission, allowing developers to implement custom functionality.
By leveraging the event object in jQuery, developers can enhance their event handling capabilities and create more interactive and dynamic web applications. Understanding how to access and utilize the information provided by the event object is essential for advanced event handling with jQuery.
In the next section, we will explore event delegation, which is another crucial technique for handling events efficiently in jQuery.
Managing Event Propagation
Managing Event Propagation
When it comes to advanced event handling with jQuery, one important concept to understand is event propagation. Event propagation refers to how events are propagated and handled in the DOM tree. By default, when an event occurs on an element, it triggers the event handlers attached to that element and then propagates to its parent elements. However, jQuery provides methods to manage event propagation and control how events are handled.
The `stopPropagation()` method in jQuery allows developers to stop the event from further propagating to parent elements. By calling this method within an event handler, developers can prevent the event from triggering any additional event handlers on parent elements. This is useful when you want to handle an event on a specific element without affecting its parent elements.
On the other hand, the `stopImmediatePropagation()` method goes a step further and not only stops the event from propagating to parent elements but also prevents any other event handlers on the current element from being executed. This method is helpful when you want to completely halt the event propagation chain and ensure that only a specific event handler is executed.
In addition to managing event propagation, jQuery also provides the `preventDefault()` method to prevent the default action associated with an event. This method is often used to override the default behavior of certain events, such as preventing a form submission or a hyperlink navigation. By calling `preventDefault()` within an event handler, developers can implement custom functionality and control the behavior of the event.
By understanding and utilizing event propagation and the methods provided by jQuery, developers can have greater control over how events are handled in their web applications. This allows for more fine-grained event management and the ability to create customized and interactive user experiences.
In the next section, we will delve into another aspect of advanced event handling with jQuery: event delegation. Event delegation is a technique that enables developers to handle events on dynamically created or modified elements efficiently. Stay tuned to explore this powerful event handling technique.
Enhancing User Experience with Event Editing
Enhancing User Experience with Event Editing
Event handling is a crucial aspect of web development, allowing websites to respond dynamically to user interactions. With jQuery’s advanced event handling capabilities, developers can easily manage and respond to user actions, enhancing the overall user experience.
One way to enhance the user experience is through event editing. By utilizing advanced event handling techniques with jQuery, developers can create interactive and dynamic web applications. This involves attaching event handlers to HTML elements and defining the actions to be performed when an event occurs.
With jQuery’s powerful event methods, developers can listen for specific user actions such as clicks, hovers, submits, and keydowns. These methods can be combined with selectors and event delegation to efficiently handle events and improve performance.
Event delegation is a technique that allows developers to handle events on dynamically created or modified elements. Instead of attaching event handlers to each individual element, a single event handler can be attached to a parent element. This improves efficiency by reducing the number of event handlers and ensuring that new elements added to the DOM still trigger the event.
In addition to event delegation, jQuery offers other advanced event handling features such as event namespacing, event bubbling, and event stopping. These features provide further control and flexibility in managing events on web pages.
By utilizing advanced event handling techniques with jQuery, developers can create web applications that provide a seamless and engaging user experience. By enhancing event editing and utilizing the power of jQuery, web developers can take their applications to the next level.
Optimizing Event Handling Performance
Optimizing Event Handling Performance
When it comes to developing web applications with advanced event handling using jQuery, optimizing performance is essential. By employing certain strategies, developers can ensure that event handling is efficient and responsive, providing a smooth user experience.
One way to optimize event handling performance is through event delegation. Rather than attaching event handlers to each individual element, event delegation allows developers to attach a single event handler to a parent element. This approach reduces the number of event handlers and improves performance, especially when dealing with dynamically created or modified elements.
Another technique to enhance performance is by utilizing event throttling and debouncing. Throttling limits the frequency of event triggers, preventing excessive event handling. Debouncing, on the other hand, delays the execution of event handlers until a certain period of inactivity has passed. These techniques are particularly useful when handling events that may trigger rapidly, such as scrolling or resizing.
Furthermore, optimizing event handling performance can be achieved by avoiding unnecessary event binding. By selectively binding event handlers only when needed and unbinding them when no longer necessary, developers can reduce the overhead associated with event handling.
Implementing efficient event handling also involves using event namespaces. Event namespaces provide a way to organize and manage related event handlers. By grouping event handlers under specific namespaces, developers can easily enable or disable them as needed, improving performance by reducing the number of active event handlers.
Lastly, it is crucial to prioritize event delegation over direct event binding for improved performance. By attaching event handlers to parent elements instead of individual elements, event delegation minimizes the number of event handlers and optimizes the event handling process.
In conclusion, when working with advanced event handling in jQuery, optimizing performance is paramount. By employing techniques such as event delegation, throttling, debouncing, and event namespaces, developers can ensure that event handling is efficient and responsive. These strategies not only enhance the user experience but also improve the overall performance of web applications.
Conclusion
In the world of web development, event handling plays a crucial role in creating dynamic and interactive user experiences. With jQuery‘s advanced event handling capabilities, developers are empowered to take their web applications to the next level. By utilizing jQuery’s powerful event methods and techniques like event delegation, event object manipulation, and event propagation management, developers can efficiently handle user interactions and enhance the overall user experience. Additionally, jQuery allows for the creation of custom events, providing developers with the flexibility to tailor event handling to their specific needs. Furthermore, optimizing event handling performance through strategies like debouncing-throttling-explained-examples/” target=”_blank”>event throttling, debouncing, and event binding management ensures that web applications perform efficiently and respond seamlessly to user actions. With the power of advanced event handling in jQuery, developers can create highly interactive and responsive web applications that engage users and elevate their browsing experience.