Generated by Contentify AI
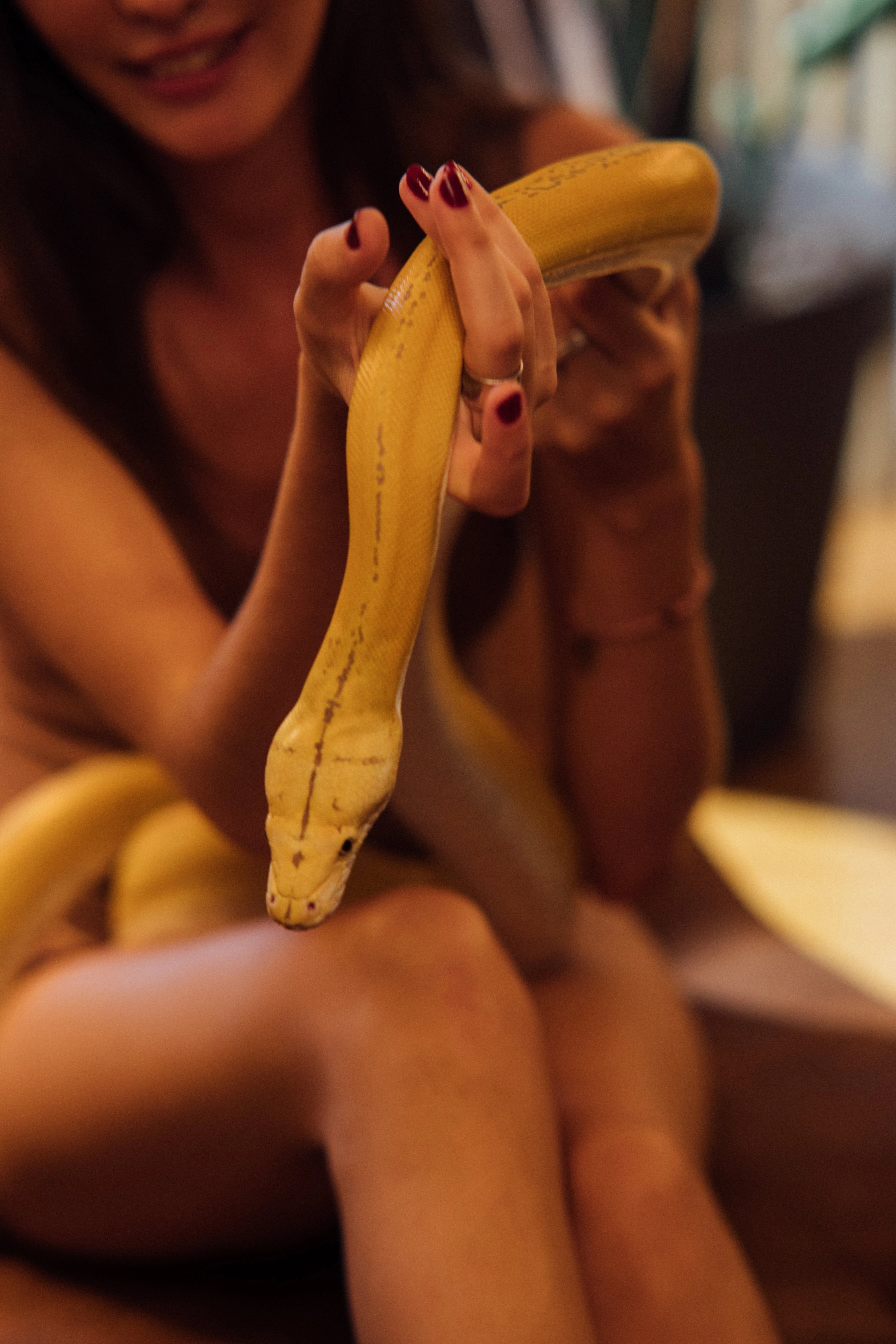
Introduction
Python for loops are an essential tool for any programmer looking to iterate over a sequence of elements or perform a certain set of instructions repeatedly. In this comprehensive guide, we will explore the various aspects of Python for loops, including their syntax, usage, and common scenarios where they can be effectively utilized. By the end of this guide, you will have a solid understanding of how to leverage for loops to streamline your coding process and solve complex problems efficiently. So, let’s dive in and explore the power of Python for loops.
Understanding Python Loops
Python for loops are a fundamental concept in programming that allow developers to iterate over a sequence of elements or execute a block of code repeatedly. With their simple syntax and versatile functionality, for loops are an essential tool in a programmer’s arsenal. By understanding how Python for loops work, you can efficiently process data, perform calculations on collections, and automate repetitive tasks. In this section, we will explore the intricacies of Python for loops, including their syntax, iteration techniques, and common use cases. By the end of this comprehensive guide, you will have a solid understanding of how to leverage Python for loops to enhance your coding productivity and solve complex problems effectively. So, let’s delve into the world of Python for loops and explore their power and versatility.
The Basics of for Loops
Python for loops are a fundamental concept in programming and a powerful tool for iterating over a sequence of elements or executing a block of code repeatedly. In this comprehensive guide to Python for loops, we will explore the basics of how for loops work and how to use them effectively in your code.
The syntax of a for loop in Python is straightforward. It starts with the keyword “for,” followed by a variable that represents each element in the sequence. Next, we use the keyword “in” to specify the sequence itself. Finally, we provide the block of code that should be executed for each element in the sequence.
For example, consider a list of numbers: [1, 2, 3, 4, 5]. To iterate over each number in the list, we can use a for loop:
“`
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
“`
In this case, the variable “num” represents each number in the list, and the print statement is executed for each element in the sequence.
For loops can also be used with other types of sequences, such as strings, tuples, or dictionaries. The key is to understand the structure of the sequence and how to access its elements within the loop.
Additionally, for loops can be used with the range() function to generate a sequence of numbers. This is useful when you need to perform a certain action a specific number of times.
“`
for i in range(5):
print(“Hello, world!”)
“`
In this example, the for loop will iterate five times, printing “Hello, world!” on each iteration.
Python for loops are versatile and can be used in various scenarios. They are commonly used for tasks such as iterating over database records, processing files, or performing calculations on collections of data.
In conclusion, understanding the basics of Python for loops is essential for any programmer. They provide a powerful and efficient way to iterate over sequences of elements and automate repetitive tasks. By mastering the syntax and techniques of for loops, you can enhance your coding productivity and solve complex problems effectively.
Iterating over Lists and Tuples
Python for loops are an essential tool for any programmer looking to iterate over a sequence of elements or perform a certain set of instructions repeatedly. In this comprehensive guide to Python for loops, we will delve into the intricacies of iterating over lists and tuples.
When it comes to working with lists and tuples, Python for loops provide a simple and efficient solution. With the ability to iterate over each element in the sequence, for loops allow you to access and process the data stored in these data structures.
To iterate over a list or tuple, you can use the same syntax as a regular for loop. For example, let’s say we have a list of names:
“`
names = [“Alice”, “Bob”, “Charlie”, “David”]
for name in names:
print(name)
“`
In this example, the variable “name” represents each element in the names list, and the print statement is executed for each element. This allows you to easily perform any desired operations on the elements within the loop.
Python for loops also provide the flexibility to combine them with other programming concepts, such as conditional statements and functions. This allows you to filter and manipulate the elements of a list or tuple based on specific conditions or apply custom operations.
Additionally, for loops can be used with the built-in range() function to generate a sequence of numbers. This can be particularly useful when working with indexes in lists or tuples.
In conclusion, Python for loops are a powerful tool for iterating over lists and tuples. They provide a flexible and efficient way to access and process the data stored in these data structures. By mastering the usage of for loops, you can streamline your code and perform complex operations on lists and tuples with ease.
Looping through Strings
Looping through Strings
One of the key applications of Python for loops is the ability to loop through strings. This allows you to process individual characters or substrings within a string. By leveraging the power of for loops, you can perform various operations on strings, such as counting occurrences, extracting information, or transforming the data.
To loop through a string, you can use the same syntax as a regular for loop. For example, let’s consider a string “Hello, World!” and iterate over each character in the string:
“`python
string = “Hello, World!”
for char in string:
print(char)
“`
In this example, the variable “char” represents each character in the string, and the print statement is executed for each character. This allows you to access and process individual characters within the loop.
Additionally, for loops can be combined with conditional statements to filter or manipulate specific characters based on certain conditions. This enables you to perform more complex operations on strings.
Furthermore, you can also use the range() function to loop through a string by index. This is helpful when you need to access and manipulate characters at specific positions within the string.
In conclusion, Python for loops provide an efficient way to loop through strings and perform operations on individual characters or substrings. By mastering the usage of for loops with strings, you can manipulate and process string data effectively in your code.
Looping through Dictionaries
Looping through Dictionaries
Dictionaries are a powerful data structure in Python that allow you to store key-value pairs. When it comes to iterating over dictionaries, Python for loops provide a convenient way to access and process the keys, values, or both simultaneously.
To loop through a dictionary, you can use the same syntax as a regular for loop. However, instead of iterating over the elements of the dictionary directly, you can use the built-in methods `keys()`, `values()`, or `items()` to access the keys, values, or both respectively.
For example, let’s consider a dictionary representing the population of different cities:
“`python
population = {
“New York City”: 8622698
,”Los Angeles”: 3990456
,”Chicago”: 2705994
,”Houston”: 2325502
}
for city in population.keys():
print(city)
“`
In this example, we use the `keys()` method to iterate over the keys of the dictionary. The variable `city` represents each key, and the print statement is executed for each key in the dictionary.
You can also loop through the values of a dictionary by using the `values()` method:
“`python
for population in population.values():
print(population)
“`
In this case, the variable `population` represents each value in the dictionary, and the print statement is executed for each value.
If you need to access both the keys and values simultaneously, you can use the `items()` method:
“`python
for city, population in population.items():
print(city, population)
“`
In this example, the variables `city` and `population` represent the key-value pairs of the dictionary, and the print statement is executed for each key-value pair.
Python for loops provide a flexible way to loop through dictionaries and process their elements. By understanding how to access the keys, values, or both, you can leverage the power of dictionaries and efficiently manipulate and analyze data stored within them.
In conclusion, Python for loops offer a comprehensive approach to looping through dictionaries. By combining the syntax of for loops with the built-in methods of dictionaries, you can access and process the keys, values, or key-value pairs. Mastery of these techniques will enable you to effectively work with dictionaries and extract valuable information from your data.
Nested Loops and Loop Control Statements
Nested Loops and Loop Control Statements
In addition to basic iteration, Python for loops offer more advanced features such as nested loops and loop control statements. These features enhance the versatility and control of for loops, allowing programmers to tackle complex problems more efficiently.
Nested loops refer to the practice of placing one loop inside another loop. This technique enables you to iterate over multiple sequences simultaneously or perform multi-dimensional operations. For example, if you have a list of names and a list of ages, you can use nested loops to iterate over both lists and match each name with its corresponding age.
“`python
names = [“Alice”, “Bob”, “Charlie”]
ages = [25, 30, 35]
for name in names:
for age in ages:
print(name, age)
“`
In this example, the outer loop iterates over the names list, while the inner loop iterates over the ages list. As a result, each name is paired with every age, producing the desired output.
Loop control statements, such as `break` and `continue`, provide additional control over the flow of a loop. With the `break` statement, you can prematurely exit a loop if a specified condition is met. This is useful when you want to stop iterating once a certain criteria is fulfilled.
“`python
names = [“Alice”, “Bob”, “Charlie”, “David”]
for name in names:
if name == “Charlie”:
break
print(name)
“`
In this example, the loop will terminate when the name “Charlie” is encountered, and the subsequent names will not be printed.
On the other hand, the `continue` statement allows you to skip the remaining code within a loop and proceed to the next iteration. This is helpful when you want to skip certain elements based on specific conditions.
“`python
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num % 2 == 0:
continue
print(num)
“`
In this case, the loop will skip even numbers and only print the odd numbers.
By incorporating nested loops and loop control statements into your Python for loops, you can solve more intricate problems and gain greater control over the flow of your code. These advanced features are valuable tools for any programmer seeking to optimize their code and enhance the efficiency of their solutions.
Common Mistakes and Best Practices
Common Mistakes and Best Practices
When working with Python for loops, it’s important to be aware of common mistakes and follow best practices to ensure efficient and error-free code. By avoiding these mistakes and following best practices, you can optimize the performance of your loops and enhance your overall coding experience.
One common mistake is not initializing variables before using them in a for loop. It is crucial to initialize any variables that you plan to use within the loop to prevent unexpected behavior or errors. Failure to initialize variables can lead to incorrect results or even code crashes.
Another mistake is modifying the sequence being iterated over within the loop. Modifying the sequence can alter the number of elements or change the order of iteration, resulting in unpredictable outcomes. To avoid this, it is recommended to create a copy of the sequence if you need to modify it while iterating.
Using excessive nested loops is another mistake to be cautious of. While nested loops can be useful, too many levels of nesting can make the code difficult to read and debug. It is important to consider alternative approaches, such as using list comprehensions or utilizing built-in functions, to simplify your code and improve its readability.
In addition to avoiding common mistakes, there are several best practices to keep in mind when working with Python for loops. Firstly, it is recommended to use meaningful variable names. Choosing descriptive and intuitive variable names improves code readability and makes it easier for others (and your future self!) to understand your code.
Another best practice is to break down complex tasks into smaller, manageable steps. By splitting a complicated task into smaller subtasks, you can approach the problem more systematically and make your code more modular and maintainable. This approach also helps with debugging and troubleshooting.
Furthermore, it is important to consider the efficiency of your loops, especially when dealing with large datasets. Using techniques such as list comprehensions or generator expressions can often provide a more efficient alternative to traditional for loops. These techniques can help optimize the memory usage and speed up the execution time of your code.
Lastly, it is crucial to test and validate your code. Before implementing your for loops in a production environment, it is recommended to test them with various inputs, including edge cases, to ensure they produce the expected results. By thorough testing and validation, you can catch and fix any potential issues before they cause problems down the line.
In conclusion, understanding the common mistakes to avoid and following best practices when working with Python for loops can greatly enhance your coding experience. By practicing good coding habits
Advanced Concepts and Examples
Advanced Concepts and Examples
Python for loops are a powerful tool for iterating over a sequence of elements or performing a set of instructions repeatedly. In this section, we will explore some advanced concepts and examples that will further enhance your understanding and usage of Python for loops.
One advanced concept is nested loops, which involve placing one loop inside another. This technique allows you to iterate over multiple sequences simultaneously or perform multi-dimensional operations. For example, you can use nested loops to match each name in a list with its corresponding age in another list.
Another advanced concept is the use of loop control statements. These statements, such as break and continue, provide additional control over the flow of a loop. The break statement allows you to prematurely exit a loop if a certain condition is met, while the continue statement skips the remaining code within a loop and moves to the next iteration.
To further illustrate these concepts, let’s consider an example where we have a list of numbers and we want to find the first even number greater than 10. We can use a for loop with a break statement to achieve this:
“`
numbers = [5, 8, 12, 15, 18, 20]
for num in numbers:
if num > 10 and num % 2 == 0:
print(num)
break
“`
In this example, the loop will iterate over the numbers list and check if each number is greater than 10 and even. Once it finds the first number that meets these conditions (in this case, 12), it will print it and exit the loop using the break statement.
Python for loops offer a wide range of possibilities and can be used in various scenarios. Whether it’s processing data, iterating over strings or dictionaries, or solving complex problems, for loops provide a versatile and efficient solution.
In conclusion, this guide has covered some advanced concepts and examples related to Python for loops. By understanding nested loops and loop control statements, you can tackle more complex problems and gain greater control over the flow of your code. Additionally, the provided example demonstrates how these concepts can be applied in real-world scenarios. With this knowledge, you can confidently utilize Python for loops to streamline your coding process and solve a wide range of problems effectively.
Conclusion
Python for loops are a powerful tool for iterating over a sequence of elements or performing a set of instructions repeatedly. In this comprehensive guide, we have explored the basics of Python for loops, including their syntax, iteration techniques, and common use cases. We have also delved into more advanced concepts such as nested loops and loop control statements, which allow for more complex operations and greater control over the flow of a loop. Additionally, we have provided examples that demonstrate the practical application of these concepts. By understanding and mastering Python for loops, you can enhance your coding productivity and solve complex problems efficiently.